Python入门基础教程
时间: 2023-11-05 08:55:49 浏览: 45
欢迎学习Python入门基础教程!下面是一些基础知识和示例代码,希望能够帮助你快速入门Python。
### 安装Python
首先,你需要在计算机上安装Python。你可以从官方网站 https://www.python.org/downloads/ 下载并安装Python 3.x版本。
### 变量和数据类型
Python中的变量不需要事先声明,可以直接赋值。例如:
```
x = 5
y = "Hello, world!"
```
Python支持多种数据类型,包括数字、字符串、列表、元组、字典等。例如:
```
# 数字
x = 5
y = 3.14
# 字符串
message = "Hello, world!"
# 列表
fruits = ["apple", "banana", "cherry"]
# 元组
colors = ("red", "green", "blue")
# 字典
person = {"name": "Alice", "age": 25}
```
### 条件语句和循环语句
Python中的条件语句和循环语句与其他编程语言类似。例如:
```
# 条件语句
x = 5
if x > 0:
print("x is positive")
elif x < 0:
print("x is negative")
else:
print("x is zero")
# 循环语句
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
i = 0
while i < 5:
print(i)
i += 1
```
### 函数
Python中的函数可以接收参数并返回值。例如:
```
def square(x):
return x * x
result = square(5)
print(result)
```
### 文件操作
Python中可以通过open函数打开文件并对其进行读写操作。例如:
```
# 打开文件
f = open("example.txt", "w")
# 写入数据
f.write("Hello, world!")
# 关闭文件
f.close()
# 读取文件
f = open("example.txt", "r")
data = f.read()
print(data)
# 关闭文件
f.close()
```
以上就是Python入门基础教程的部分内容,希望能够帮助你快速入门Python。
相关推荐
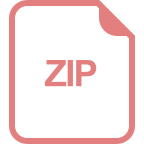
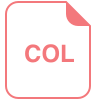
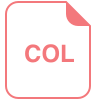
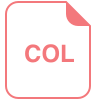
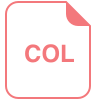
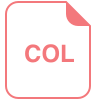









