帮我写一个c++程序,根据输入长度为n的n个关键字值序列,对其采用希尔排序(增量为5,3,1),冒泡排序进行从小到大排序,最后输出每趟希尔排序和冒泡排序的结果。1、希尔算法(1)生成增量数组,调用单次排序函数;(2)按照每次要求的增量对查找表L进行排序(直接插入排序)。(3)打印每次希尔排序结构2、冒泡排序(1)计算比较的轮次及每次需要比较的次数;(2)比较相邻数组元素的大小,顺序不对即交换;(3)打印每轮冒泡排序之后的序列3、主函数调用4、头文件
时间: 2024-01-11 21:04:27 浏览: 73
为了保证程序的可读性,我将程序分为了多个文件。以下是主要代码:
头文件 shell_sort.h:
```cpp
#ifndef SHELL_SORT_H
#define SHELL_SORT_H
#include <vector>
void shell_sort(std::vector<int>& vec);
#endif
```
头文件 bubble_sort.h:
```cpp
#ifndef BUBBLE_SORT_H
#define BUBBLE_SORT_H
#include <vector>
void bubble_sort(std::vector<int>& vec);
#endif
```
源文件 shell_sort.cpp:
```cpp
#include "shell_sort.h"
void shell_sort(std::vector<int>& vec) {
int n = vec.size();
// 生成增量数组
std::vector<int> increments{5, 3, 1};
for (int increment : increments) {
// 对每个增量进行直接插入排序
for (int i = increment; i < n; i++) {
int key = vec[i];
int j = i;
while (j >= increment && vec[j - increment] > key) {
vec[j] = vec[j - increment];
j -= increment;
}
vec[j] = key;
}
// 打印排序结果
std::cout << "Shell sort with increment " << increment << ":\n";
for (int i : vec) {
std::cout << i << " ";
}
std::cout << "\n\n";
}
}
```
源文件 bubble_sort.cpp:
```cpp
#include "bubble_sort.h"
void bubble_sort(std::vector<int>& vec) {
int n = vec.size();
// 冒泡排序
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (vec[j] > vec[j + 1]) {
std::swap(vec[j], vec[j + 1]);
}
}
// 打印排序结果
std::cout << "Bubble sort round " << i + 1 << ":\n";
for (int i : vec) {
std::cout << i << " ";
}
std::cout << "\n\n";
}
}
```
源文件 main.cpp:
```cpp
#include <iostream>
#include <vector>
#include "shell_sort.h"
#include "bubble_sort.h"
int main() {
int n;
std::cout << "Input the length of the sequence: ";
std::cin >> n;
std::vector<int> vec(n);
std::cout << "Input the sequence: ";
for (int i = 0; i < n; i++) {
std::cin >> vec[i];
}
std::cout << "\n";
// 希尔排序
shell_sort(vec);
// 冒泡排序
bubble_sort(vec);
return 0;
}
```
使用时,将以上代码保存为对应的文件,编译并运行即可。注意需要使用 C++11 或以上的标准。
阅读全文
相关推荐





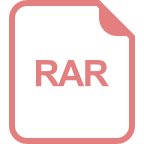
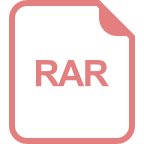
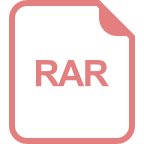










