帮我用c语言和easyx图形库写一个华容道小游戏的完整代码
时间: 2024-05-15 09:14:27 浏览: 13
好的,以下是一个简单的华容道小游戏的完整代码,使用了C语言和EasyX图形库:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROW 4 // 行数
#define COL 4 // 列数
#define SIZE 100 // 每个方块的大小
#define MARGIN 20 // 边距
#define WIDTH COL*SIZE+MARGIN*2 // 窗口宽度
#define HEIGHT ROW*SIZE+MARGIN*2 // 窗口高度
int map[ROW][COL]; // 地图数组,用来记录每个方块的状态
int empty_x, empty_y; // 空白方块的坐标
// 初始化地图
void init_map()
{
int i, j;
int num = 1;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
map[i][j] = num++;
}
}
map[ROW - 1][COL - 1] = 0;
empty_x = ROW - 1;
empty_y = COL - 1;
}
// 绘制地图
void draw_map()
{
int i, j;
setbkcolor(WHITE);
cleardevice();
settextcolor(BLACK);
settextstyle(40, 0, "宋体");
outtextxy(MARGIN, MARGIN / 2, "华容道小游戏");
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (map[i][j]) {
// 绘制方块
setfillcolor(BLUE);
setlinecolor(BLACK);
fillrectangle(j*SIZE + MARGIN, i*SIZE + MARGIN, (j+1)*SIZE + MARGIN, (i+1)*SIZE + MARGIN);
char str[10];
sprintf(str, "%d", map[i][j]);
settextstyle(40, 0, "宋体");
settextcolor(WHITE);
outtextxy(j*SIZE + MARGIN + SIZE / 2 - 10, i*SIZE + MARGIN + SIZE / 2 - 20, str);
}
else {
empty_x = i;
empty_y = j;
}
}
}
}
// 移动方块
void move(int x, int y)
{
int temp;
if ((x == empty_x && abs(y - empty_y) == 1) || (y == empty_y && abs(x - empty_x) == 1)) {
temp = map[x][y];
map[x][y] = map[empty_x][empty_y];
map[empty_x][empty_y] = temp;
empty_x = x;
empty_y = y;
}
}
// 判断游戏是否结束
int is_win()
{
int i, j;
int num = 1;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (map[i][j] != num++) {
return 0;
}
}
}
return 1;
}
int main()
{
initgraph(WIDTH, HEIGHT);
init_map();
draw_map();
// 随机打乱地图
srand((unsigned)time(NULL));
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
int x = rand() % ROW;
int y = rand() % COL;
int temp = map[i][j];
map[i][j] = map[x][y];
map[x][y] = temp;
}
}
draw_map();
while (!is_win()) {
if (kbhit()) {
char ch = getch();
switch (ch) {
case 'w':
move(empty_x - 1, empty_y);
break;
case 's':
move(empty_x + 1, empty_y);
break;
case 'a':
move(empty_x, empty_y - 1);
break;
case 'd':
move(empty_x, empty_y + 1);
break;
default:
break;
}
draw_map();
}
}
// 游戏结束,显示提示信息
settextstyle(40, 0, "宋体");
settextcolor(RED);
outtextxy(MARGIN, HEIGHT - MARGIN, "恭喜你,游戏结束!");
getch();
closegraph();
return 0;
}
```
该代码实现了一个简单的华容道小游戏,可以通过键盘控制方块的移动,直到完成游戏。游戏结束后会显示提示信息。
相关推荐
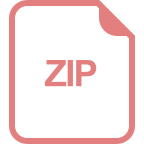
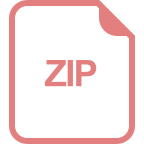














