在datagirdview中将数据显示到textbox中并在textbox中修改数据通过button修改数据库
时间: 2024-03-16 11:42:26 浏览: 16
好的,让我来解答你的问题。
首先,你需要将 DataGridView 绑定到数据源,这可以通过设置 DataSource 属性来实现。然后,在 DataGridView 中选中要编辑的行,将其数据加载到 TextBox 中,以便进行修改。
接下来,你可以创建一个保存按钮,当用户点击该按钮时,将 TextBox 中的修改保存回数据库。在保存之前,你需要获取 TextBox 中的值并将其与 DataGridView 中的相应行和列对应。
以下是一些示例代码,演示如何在 DataGridView 和 TextBox 之间进行数据传输,并将修改保存回数据库。
```csharp
// 将数据显示在 DataGridView 中
private void LoadData()
{
string connectionString = "your_connection_string_here";
string query = "SELECT * FROM your_table_here";
DataTable data = new DataTable();
using (SqlConnection connection = new SqlConnection(connectionString))
using (SqlCommand command = new SqlCommand(query, connection))
using (SqlDataAdapter adapter = new SqlDataAdapter(command))
{
adapter.Fill(data);
}
dataGridView1.DataSource = data;
}
// 将选中的行数据显示在 TextBox 中
private void dataGridView1_SelectionChanged(object sender, EventArgs e)
{
if (dataGridView1.SelectedRows.Count > 0)
{
DataGridViewRow row = dataGridView1.SelectedRows[0];
textBox1.Text = row.Cells["column_name_here"].Value.ToString();
}
}
// 保存修改回数据库
private void btnSave_Click(object sender, EventArgs e)
{
if (dataGridView1.SelectedRows.Count > 0)
{
DataGridViewRow row = dataGridView1.SelectedRows[0];
string id = row.Cells["id_column_name_here"].Value.ToString();
string newValue = textBox1.Text;
string connectionString = "your_connection_string_here";
string query = "UPDATE your_table_here SET column_name_here = @newValue WHERE id_column_name_here = @id";
using (SqlConnection connection = new SqlConnection(connectionString))
using (SqlCommand command = new SqlCommand(query, connection))
{
command.Parameters.AddWithValue("@newValue", newValue);
command.Parameters.AddWithValue("@id", id);
connection.Open();
command.ExecuteNonQuery();
}
MessageBox.Show("修改已保存!");
}
}
```
希望这些代码能帮助到你完成你的需求。如果你还有任何问题,请随时问我。
相关推荐
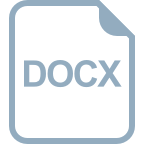
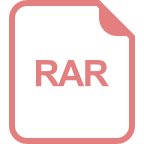














