python实现des
时间: 2023-08-31 09:38:39 浏览: 79
### 回答1:
DES(Data Encryption Standard)是一种对称密钥加密算法,它的密钥长度为 56 位,分为 8 个字节。下面是 Python 实现 DES 的示例代码:
```python
from Crypto.Cipher import DES
from Crypto.Util.Padding import pad, unpad
def des_encrypt(message, key):
cipher = DES.new(key, DES.MODE_ECB)
padded_message = pad(message.encode(), DES.block_size)
encrypted_message = cipher.encrypt(padded_message)
return encrypted_message
def des_decrypt(encrypted_message, key):
cipher = DES.new(key, DES.MODE_ECB)
decrypted_message = cipher.decrypt(encrypted_message)
unpadded_message = unpad(decrypted_message, DES.block_size)
return unpadded_message.decode()
# 测试
key = b'abcdefgh'
message = 'hello world'
encrypted_message = des_encrypt(message, key)
print(encrypted_message)
decrypted_message = des_decrypt(encrypted_message, key)
print(decrypted_message)
```
在这个示例中,我们使用了 PyCrypto 库(现在已经不再维护,可以使用 Crypto 库代替),它提供了 DES 的实现。我们首先定义了两个函数 `des_encrypt` 和 `des_decrypt`,前者用于加密消息,后者用于解密消息。在加密和解密时,我们都要使用相同的密钥 `key`。
在加密时,我们首先创建一个 DES 对象 `cipher`,指定加密模式为 ECB(电子密码本模式)。然后,我们使用 `pad` 函数将消息 `message` 进行填充,使其长度是 DES 的分组大小(8 个字节)。接着,我们使用 `encrypt` 函数对填充后的消息进行加密,并返回密文。
在解密时,我们首先创建一个 DES 对象 `cipher`,指定加密模式为 ECB。然后,我们使用 `decrypt` 函数对密文 `encrypted_message` 进行解密,得到填充后的消息。最后,我们使用 `unpad` 函数将填充去除,并将消息转换为字符串,返回明文。
### 回答2:
DES(Data Encryption Standard)是一种对称加密算法,常用于数据加密和解密领域。Python提供了多种实现DES算法的方式,其中最常用的是使用pycryptodome库。
要实现DES算法,首先需要安装pycryptodome库。可以使用pip安装命令进行安装:pip install pycryptodome。
接下来,可以使用下面的代码实现DES加密和解密:
```python
from Crypto.Cipher import DES
from Crypto.Random import get_random_bytes
import base64
# 生成随机的8字节密钥
key = get_random_bytes(8)
# 明文需要为8字节的倍数
plaintext = b'This is a secret message'
# 创建DES对象并进行加密
cipher = DES.new(key, DES.MODE_ECB)
ciphertext = cipher.encrypt(plaintext)
# 将加密后的密文进行Base64编码
encoded_ciphertext = base64.b64encode(ciphertext)
# 输出加密后的结果
print("加密后的密文:", encoded_ciphertext)
# 创建新的DES对象并进行解密
decipher = DES.new(key, DES.MODE_ECB)
decoded_ciphertext = base64.b64decode(encoded_ciphertext)
decrypted_text = decipher.decrypt(decoded_ciphertext)
# 输出解密后的结果
print("解密后的明文:", decrypted_text)
```
该代码首先生成一个随机的8字节密钥,然后创建DES对象并指定使用ECB模式进行加密。将明文加密后,使用Base64进行编码以便输出结果。然后,创建新的DES对象并使用相同的密钥进行解密。最后输出解密后的明文。
这样,就完成了使用Python实现DES算法的过程。当然,在实际应用中,需要注意密钥的安全和密文的传输等问题。
相关推荐
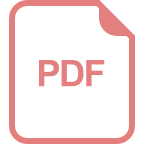
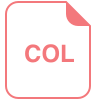
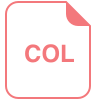
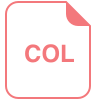










