请用Java写出通过顺序存储结构和链式存储结构构建顺序表、链表、顺序栈、链栈的代码(学生属性包括:序号、学号、姓名、性别、年龄、班级)
时间: 2023-07-04 10:04:32 浏览: 55
顺序存储结构构建顺序表:
```
public class Student {
int id; // 序号
String no; // 学号
String name; // 姓名
String gender; // 性别
int age; // 年龄
String clazz; // 班级
public Student(int id, String no, String name, String gender, int age, String clazz) {
this.id = id;
this.no = no;
this.name = name;
this.gender = gender;
this.age = age;
this.clazz = clazz;
}
}
public class SeqList {
private Student[] data; // 存储元素的数组
private int size; // 当前元素个数
private int capacity; // 容量
public SeqList(int capacity) {
this.data = new Student[capacity];
this.size = 0;
this.capacity = capacity;
}
// 获取指定位置的元素
public Student get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException();
}
return data[index];
}
// 插入元素到指定位置
public void insert(int index, Student student) {
if (index < 0 || index > size) {
throw new IndexOutOfBoundsException();
}
if (size == capacity) {
throw new RuntimeException("SeqList is full.");
}
for (int i = size - 1; i >= index; i--) {
data[i + 1] = data[i];
}
data[index] = student;
size++;
}
// 删除指定位置的元素
public void delete(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException();
}
for (int i = index; i < size - 1; i++) {
data[i] = data[i + 1];
}
data[size - 1] = null;
size--;
}
// 获取元素个数
public int size() {
return size;
}
// 判断是否为空
public boolean isEmpty() {
return size == 0;
}
}
```
链式存储结构构建链表:
```
public class Student {
int id; // 序号
String no; // 学号
String name; // 姓名
String gender; // 性别
int age; // 年龄
String clazz; // 班级
public Student(int id, String no, String name, String gender, int age, String clazz) {
this.id = id;
this.no = no;
this.name = name;
this.gender = gender;
this.age = age;
this.clazz = clazz;
}
}
public class ListNode {
Student student;
ListNode next;
public ListNode(Student student) {
this.student = student;
this.next = null;
}
}
public class LinkedList {
private ListNode head; // 头结点
private int size; // 当前元素个数
public LinkedList() {
this.head = null;
this.size = 0;
}
// 在链表头部插入元素
public void insertAtHead(Student student) {
ListNode newNode = new ListNode(student);
newNode.next = head;
head = newNode;
size++;
}
// 在链表尾部插入元素
public void insertAtTail(Student student) {
ListNode newNode = new ListNode(student);
if (head == null) {
head = newNode;
} else {
ListNode p = head;
while (p.next != null) {
p = p.next;
}
p.next = newNode;
}
size++;
}
// 在指定位置插入元素
public void insert(int index, Student student) {
if (index < 0 || index > size) {
throw new IndexOutOfBoundsException();
}
if (index == 0) {
insertAtHead(student);
} else if (index == size) {
insertAtTail(student);
} else {
ListNode newNode = new ListNode(student);
ListNode p = head;
for (int i = 0; i < index - 1; i++) {
p = p.next;
}
newNode.next = p.next;
p.next = newNode;
size++;
}
}
// 删除指定位置的元素
public void delete(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException();
}
if (index == 0) {
head = head.next;
} else {
ListNode p = head;
for (int i = 0; i < index - 1; i++) {
p = p.next;
}
p.next = p.next.next;
}
size--;
}
// 获取元素个数
public int size() {
return size;
}
// 判断是否为空
public boolean isEmpty() {
return size == 0;
}
}
```
顺序存储结构构建顺序栈:
```
public class Student {
int id; // 序号
String no; // 学号
String name; // 姓名
String gender; // 性别
int age; // 年龄
String clazz; // 班级
public Student(int id, String no, String name, String gender, int age, String clazz) {
this.id = id;
this.no = no;
this.name = name;
this.gender = gender;
this.age = age;
this.clazz = clazz;
}
}
public class SeqStack {
private Student[] data; // 存储元素的数组
private int top; // 栈顶指针
private int capacity; // 容量
public SeqStack(int capacity) {
this.data = new Student[capacity];
this.top = -1;
this.capacity = capacity;
}
// 入栈
public void push(Student student) {
if (top == capacity - 1) {
throw new RuntimeException("SeqStack is full.");
}
data[++top] = student;
}
// 出栈
public Student pop() {
if (top == -1) {
throw new RuntimeException("SeqStack is empty.");
}
return data[top--];
}
// 获取栈顶元素
public Student peek() {
if (top == -1) {
throw new RuntimeException("SeqStack is empty.");
}
return data[top];
}
// 获取元素个数
public int size() {
return top + 1;
}
// 判断是否为空
public boolean isEmpty() {
return top == -1;
}
}
```
链式存储结构构建链栈:
```
public class Student {
int id; // 序号
String no; // 学号
String name; // 姓名
String gender; // 性别
int age; // 年龄
String clazz; // 班级
public Student(int id, String no, String name, String gender, int age, String clazz) {
this.id = id;
this.no = no;
this.name = name;
this.gender = gender;
this.age = age;
this.clazz = clazz;
}
}
public class ListNode {
Student student;
ListNode next;
public ListNode(Student student) {
this.student = student;
this.next = null;
}
}
public class LinkedStack {
private ListNode top; // 栈顶指针
public LinkedStack() {
this.top = null;
}
// 入栈
public void push(Student student) {
ListNode newNode = new ListNode(student);
newNode.next = top;
top = newNode;
}
// 出栈
public Student pop() {
if (top == null) {
throw new RuntimeException("LinkedStack is empty.");
}
Student student = top.student;
top = top.next;
return student;
}
// 获取栈顶元素
public Student peek() {
if (top == null) {
throw new RuntimeException("LinkedStack is empty.");
}
return top.student;
}
// 获取元素个数
public int size() {
int count = 0;
ListNode p = top;
while (p != null) {
count++;
p = p.next;
}
return count;
}
// 判断是否为空
public boolean isEmpty() {
return top == null;
}
}
```
相关推荐
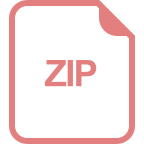
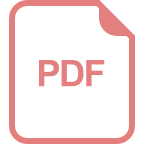
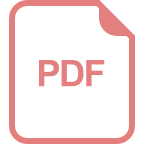














