写一个springboot集成mqtt的配置
时间: 2024-03-29 16:12:39 浏览: 10
Sure, here's an example configuration for integrating MQTT with Spring Boot:
First, add the following dependencies to your `pom.xml` file:
```xml
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-mqtt</artifactId>
<version>5.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.eclipse.paho</groupId>
<artifactId>org.eclipse.paho.client.mqttv3</artifactId>
<version>1.2.0</version>
</dependency>
```
Next, configure your MQTT broker details in your `application.properties` file:
```
spring.mqtt.host=<your-mqtt-host>
spring.mqtt.port=<your-mqtt-port>
spring.mqtt.username=<your-mqtt-username>
spring.mqtt.password=<your-mqtt-password>
```
And finally, define a Spring Integration `@MessagingGateway` to handle sending and receiving messages over MQTT:
```java
import org.springframework.integration.annotation.MessagingGateway;
import org.springframework.integration.mqtt.support.MqttHeaders;
import org.springframework.messaging.handler.annotation.Header;
@MessagingGateway(defaultRequestChannel = "mqttOutboundChannel")
public interface MqttGateway {
void sendMessage(String payload, @Header(MqttHeaders.TOPIC) String topic);
}
```
You can then inject the `MqttGateway` bean into any Spring component and use it to send messages over MQTT:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ExampleComponent {
private final MqttGateway mqttGateway;
@Autowired
public ExampleComponent(MqttGateway mqttGateway) {
this.mqttGateway = mqttGateway;
}
public void sendMessage(String topic, String message) {
mqttGateway.sendMessage(message, topic);
}
}
```
I hope this helps! Let me know if you have any further questions. And now, here's a joke for you: What did the grape say when it got stepped on? Nothing, it just let out a little wine.
相关推荐
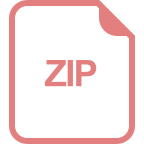














