linuxC 语言编写一个100以内加减乘除的出题库 并自动判断成绩,要求乘法 除法要完全整除
时间: 2024-03-12 09:49:33 浏览: 18
好的,这个需求可以分为两个部分来实现:
1. 生成100以内加减乘除的出题库
可以编写一个函数,使用随机数生成器生成两个随机数,再根据随机数生成一个运算符,最后将题目以字符串的形式输出。例如:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
char* generate_question() {
char* question = (char*) malloc(sizeof(char) * 10);
int a = rand() % 100 + 1;
int b = rand() % 100 + 1;
int op = rand() % 4; // 0: +, 1: -, 2: *, 3: /
switch (op) {
case 0:
sprintf(question, "%d + %d", a, b);
break;
case 1:
sprintf(question, "%d - %d", a, b);
break;
case 2:
sprintf(question, "%d * %d", a, b);
break;
case 3:
while (a % b != 0) {
a = rand() % 100 + 1;
b = rand() % 100 + 1;
}
sprintf(question, "%d / %d", a, b);
break;
}
return question;
}
```
注意,除法题目需要使用循环确保除数能够整除被除数。
2. 自动判断成绩
可以编写一个主函数,循环输出题目并等待用户输入答案,然后判断答案是否正确,最后给出总得分。例如:
```c
int main() {
srand(time(NULL)); // 初始化随机数生成器
int score = 0;
for (int i = 0; i < 10; i++) {
char* question = generate_question();
printf("Question %d: %s = ", i + 1, question);
int answer;
scanf("%d", &answer);
int a, b;
sscanf(question, "%d %*c %*c %d", &a, &b);
switch (question[strlen(question) - 1]) {
case '+':
if (answer == a + b) score++;
break;
case '-':
if (answer == a - b) score++;
break;
case '*':
if (answer == a * b) score++;
break;
case '/':
if (answer == a / b) score++;
break;
}
free(question);
}
printf("Your score is %d/10.\n", score);
return 0;
}
```
这个主函数使用了 `generate_question` 函数生成题目,并且使用 `scanf` 函数等待用户输入答案。然后根据题目中的运算符判断答案是否正确,最后给出总得分。
完整代码如下:
相关推荐
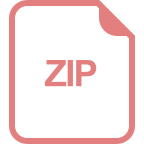














