写成c++代码
时间: 2023-07-09 21:12:09 浏览: 61
以下是一个简单的 C++ 代码示例,用于实现带有约束条件的移动最小二乘法,并让拟合曲线通过两个定点 $(x_1, y_1)$ 和 $(x_2, y_2)$:
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 定义拟合函数
double f(double x, vector<double>& theta) {
return theta[0] + theta[1] * x + theta[2] * x * x;
}
// 定义目标函数
double cost(vector<double>& x, vector<double>& y, vector<double>& theta, double x1, double y1, double x2, double y2) {
double sum = 0;
for(int i=0; i<x.size(); i++) {
sum += pow(y[i]-f(x[i], theta), 2);
}
// 加入约束条件
sum += pow(f(x1, theta)-y1, 2);
sum += pow(f(x2, theta)-y2, 2);
return sum;
}
// 定义梯度函数
vector<double> gradient(vector<double>& x, vector<double>& y, vector<double>& theta, double x1, double y1, double x2, double y2) {
vector<double> grad(theta.size(), 0);
for(int i=0; i<x.size(); i++) {
double err = y[i] - f(x[i], theta);
grad[0] += -2 * err;
grad[1] += -2 * err * x[i];
grad[2] += -2 * err * x[i] * x[i];
}
// 加入约束条件
grad[0] += 2 * (f(x1, theta) - y1);
grad[1] += 2 * (f(x1, theta) - y1) * x1;
grad[2] += 2 * (f(x1, theta) - y1) * x1 * x1;
grad[0] += 2 * (f(x2, theta) - y2);
grad[1] += 2 * (f(x2, theta) - y2) * x2;
grad[2] += 2 * (f(x2, theta) - y2) * x2 * x2;
return grad;
}
// 定义优化函数
vector<double> optimize(vector<double>& x, vector<double>& y, vector<double>& theta, double x1, double y1, double x2, double y2, double alpha, int maxIter) {
for(int iter=0; iter<maxIter; iter++) {
vector<double> grad = gradient(x, y, theta, x1, y1, x2, y2);
for(int i=0; i<theta.size(); i++) {
theta[i] -= alpha * grad[i];
}
}
return theta;
}
int main() {
// 定义数据和模型参数
vector<double> x = {1, 2, 3, 4, 5};
vector<double> y = {2, 3, 5, 6, 8};
vector<double> theta = {1, 1, 1};
double x1 = 1, y1 = 2, x2 = 5, y2 = 8;
// 调用优化函数
theta = optimize(x, y, theta, x1, y1, x2, y2, 0.01, 1000);
// 输出最终结果
cout << "theta: ";
for(int i=0; i<theta.size(); i++) {
cout << theta[i] << " ";
}
cout << endl;
return 0;
}
```
上述代码中,首先定义了拟合函数 $f(x, \theta)$,目标函数 `cost` 和梯度函数 `gradient`。其中,目标函数包括了两个约束条件,分别是让拟合曲线通过 $(x_1, y_1)$ 和 $(x_2, y_2)$ 两个点。梯度函数也相应地加入了这两个约束条件的梯度计算。
然后,在 `optimize` 函数中调用梯度下降算法进行优化,最终输出最终的模型参数 $\theta$。
需要注意的是,上述代码只是一个简单的示例,实际应用中还需要根据具体情况进行调整。
阅读全文
相关推荐
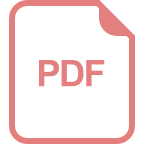
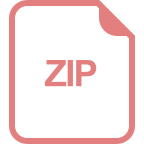
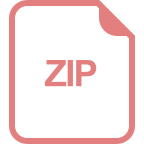
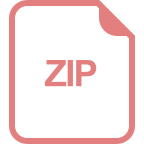
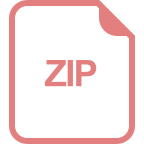
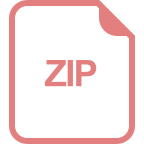
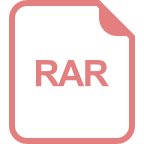
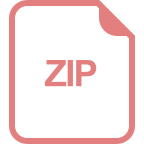
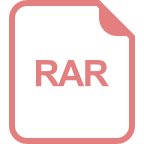
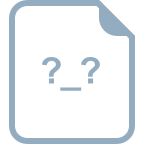
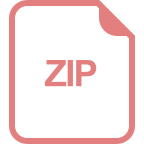
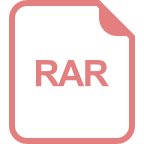
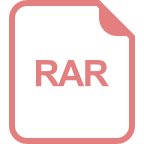
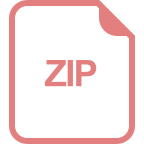
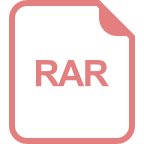