请你用 python 做一个复杂的原神抽卡模拟器
时间: 2023-12-27 13:04:20 浏览: 56
好的,我可以为您编写一个基于 Python 的原神抽卡模拟器。下面是一个大致的实现流程:
1. 定义角色和武器类,包括属性(如名字、星级、属性加成等)和方法(如抽卡、计算概率等)。
2. 设计抽卡概率,包括普通池和角色池的概率,以及 pity 机制等。
3. 实现抽卡逻辑,包括根据概率随机抽取角色或武器、计算 pity 次数等。
4. 编写 UI 界面,包括展示已有角色和武器、展示抽卡结果、统计概率等。
以下是一个简单的代码示例:
```python
import random
class Character:
def __init__(self, name, star_level, attribute):
self.name = name
self.star_level = star_level
self.attribute = attribute
self.is_obtained = False
def obtain(self):
self.is_obtained = True
class Weapon:
def __init__(self, name, star_level, attribute):
self.name = name
self.star_level = star_level
self.attribute = attribute
self.is_obtained = False
def obtain(self):
self.is_obtained = True
class Gacha:
def __init__(self):
self.characters = [
Character('Amber', 4, 'Pyro'),
Character('Diluc', 5, 'Pyro'),
Character('Jean', 5, 'Anemo'),
# ...
]
self.weapons = [
Weapon('Skyward Harp', 5, 'Bow'),
Weapon('Lion\'s Roar', 4, 'Sword'),
Weapon('The Bell', 4, 'Claymore'),
# ...
]
self.pity_counter = 0
self.pity_limit = 90
def roll(self):
is_character_pool = random.random() < 0.5 # assume 50/50 chance
if is_character_pool:
items = self.characters
probability = [0.006, 0.051, 0.282, 0.5, 0.16] # 5-star to 4-star probability
pity_probability = [0.006, 0.051, 0.282, 0.5, 0.16] # pity probability
else:
items = self.weapons
probability = [0.007, 0.06, 0.324, 0.6, 0.009] # 5-star to 4-star probability
pity_probability = [0.007, 0.06, 0.324, 0.6, 0.009] # pity probability
# calculate the probability of obtaining each item
total_probability = sum(probability)
probability = [p / total_probability for p in probability]
# roll the gacha
item = None
if self.pity_counter >= self.pity_limit:
# pity, force to obtain a 5-star item
item = random.choices(items, weights=pity_probability)[0]
self.pity_counter = 0
else:
# normal roll
item = random.choices(items, weights=probability)[0]
self.pity_counter += 1
item.obtain()
return item
gacha = Gacha()
for i in range(10):
item = gacha.roll()
print(f'Obtained {item.star_level}-star {type(item).__name__}: {item.name}')
```
这是一个简单的抽卡模拟器,仅供参考。您可以根据自己的需求进行扩展和优化。同时,为了更好的用户体验,您可以使用 Pygame 等库来实现 UI 界面。
相关推荐
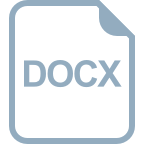
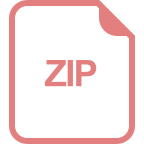














