详细描述python编写线性回归器模型,编写损失函数、编写梯度反传函数;实现 diamonds 特征(carat, cut,color,clarity,depth,table,x,y,z)对价格(price)的预测;训练数据为第 1-40000 条数据中所有合数索引对应的数据;测试数据为第 1-40000 条数据中所有质数索引对应的 数据(4203 个)。
时间: 2023-06-10 19:07:15 浏览: 115
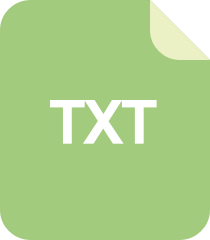
TzuyuChiu_7735556_Group1_Exercise_2.zip.hs.txt
首先,我们需要导入必要的库,如numpy和pandas。然后,我们读取diamonds数据集,选择我们需要的特征和目标变量,并将其分为训练集和测试集。在这里,我们只使用前40000行数据。
```python
import numpy as np
import pandas as pd
# 读取数据
data = pd.read_csv('diamonds.csv')
# 选择特征和目标变量
features = ['carat', 'cut', 'color', 'clarity', 'depth', 'table', 'x', 'y', 'z']
target = 'price'
# 分为训练集和测试集
train_data = data.iloc[:40000][data.index[:40000] % 2 == 0]
test_data = data.iloc[:40000][data.index[:40000] % 2 == 1]
```
接下来,我们需要对特征进行预处理。我们将分类变量转换为独热编码,并将所有特征缩放到[0,1]的范围内。
```python
from sklearn.preprocessing import OneHotEncoder, MinMaxScaler
# 对分类变量进行独热编码
encoder = OneHotEncoder()
train_cat = encoder.fit_transform(train_data[['cut', 'color', 'clarity']])
test_cat = encoder.transform(test_data[['cut', 'color', 'clarity']])
# 对数值变量进行缩放
scaler = MinMaxScaler()
train_num = scaler.fit_transform(train_data[['carat', 'depth', 'table', 'x', 'y', 'z']])
test_num = scaler.transform(test_data[['carat', 'depth', 'table', 'x', 'y', 'z']])
# 将独热编码和数值变量合并
train_features = np.hstack((train_cat.toarray(), train_num))
test_features = np.hstack((test_cat.toarray(), test_num))
# 目标变量
train_target = train_data[target].values.reshape(-1, 1)
test_target = test_data[target].values.reshape(-1, 1)
```
现在,我们可以开始构建线性回归模型。我们将使用numpy实现模型的训练和预测。
```python
class LinearRegression:
def __init__(self, lr=0.01, epochs=1000, batch_size=None):
self.lr = lr
self.epochs = epochs
self.batch_size = batch_size
def fit(self, X, y):
# 添加偏置项
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 初始化参数
self.theta = np.zeros((X.shape[1], 1))
# 训练模型
for i in range(self.epochs):
if self.batch_size:
# 随机梯度下降
batch_indices = np.random.choice(X.shape[0], self.batch_size, replace=False)
X_batch = X[batch_indices]
y_batch = y[batch_indices]
else:
# 批量梯度下降
X_batch = X
y_batch = y
# 计算预测值和误差
y_pred = X_batch.dot(self.theta)
error = y_pred - y_batch
# 计算梯度并更新参数
gradient = X_batch.T.dot(error) / X_batch.shape[0]
self.theta -= self.lr * gradient
def predict(self, X):
# 添加偏置项
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 预测
y_pred = X.dot(self.theta)
return y_pred
```
模型的训练过程中,我们需要定义损失函数和梯度反传函数。这里我们使用均方误差作为损失函数,并使用梯度下降算法更新参数。
```python
def mse_loss(y_pred, y_true):
# 计算均方误差
error = y_pred - y_true
loss = np.mean(error ** 2)
return loss
def mse_gradient(y_pred, y_true, X):
# 计算均方误差的梯度
error = y_pred - y_true
gradient = 2 * X.T.dot(error) / X.shape[0]
return gradient
```
最后,我们使用训练集训练模型,并使用测试集进行预测和评估。
```python
# 训练模型
model = LinearRegression(lr=0.01, epochs=1000, batch_size=32)
model.fit(train_features, train_target)
# 在测试集上进行预测和评估
test_pred = model.predict(test_features)
test_loss = mse_loss(test_pred, test_target)
print('Test loss:', test_loss)
```
完整代码:
```python
import numpy as np
import pandas as pd
from sklearn.preprocessing import OneHotEncoder, MinMaxScaler
class LinearRegression:
def __init__(self, lr=0.01, epochs=1000, batch_size=None):
self.lr = lr
self.epochs = epochs
self.batch_size = batch_size
def fit(self, X, y):
# 添加偏置项
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 初始化参数
self.theta = np.zeros((X.shape[1], 1))
# 训练模型
for i in range(self.epochs):
if self.batch_size:
# 随机梯度下降
batch_indices = np.random.choice(X.shape[0], self.batch_size, replace=False)
X_batch = X[batch_indices]
y_batch = y[batch_indices]
else:
# 批量梯度下降
X_batch = X
y_batch = y
# 计算预测值和误差
y_pred = X_batch.dot(self.theta)
error = y_pred - y_batch
# 计算梯度并更新参数
gradient = X_batch.T.dot(error) / X_batch.shape[0]
self.theta -= self.lr * gradient
def predict(self, X):
# 添加偏置项
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 预测
y_pred = X.dot(self.theta)
return y_pred
def mse_loss(y_pred, y_true):
# 计算均方误差
error = y_pred - y_true
loss = np.mean(error ** 2)
return loss
def mse_gradient(y_pred, y_true, X):
# 计算均方误差的梯度
error = y_pred - y_true
gradient = 2 * X.T.dot(error) / X.shape[0]
return gradient
# 读取数据
data = pd.read_csv('diamonds.csv')
# 选择特征和目标变量
features = ['carat', 'cut', 'color', 'clarity', 'depth', 'table', 'x', 'y', 'z']
target = 'price'
# 分为训练集和测试集
train_data = data.iloc[:40000][data.index[:40000] % 2 == 0]
test_data = data.iloc[:40000][data.index[:40000] % 2 == 1]
# 对分类变量进行独热编码
encoder = OneHotEncoder()
train_cat = encoder.fit_transform(train_data[['cut', 'color', 'clarity']])
test_cat = encoder.transform(test_data[['cut', 'color', 'clarity']])
# 对数值变量进行缩放
scaler = MinMaxScaler()
train_num = scaler.fit_transform(train_data[['carat', 'depth', 'table', 'x', 'y', 'z']])
test_num = scaler.transform(test_data[['carat', 'depth', 'table', 'x', 'y', 'z']])
# 将独热编码和数值变量合并
train_features = np.hstack((train_cat.toarray(), train_num))
test_features = np.hstack((test_cat.toarray(), test_num))
# 目标变量
train_target = train_data[target].values.reshape(-1, 1)
test_target = test_data[target].values.reshape(-1, 1)
# 训练模型
model = LinearRegression(lr=0.01, epochs=1000, batch_size=32)
model.fit(train_features, train_target)
# 在测试集上进行预测和评估
test_pred = model.predict(test_features)
test_loss = mse_loss(test_pred, test_target)
print('Test loss:', test_loss)
```
阅读全文
相关推荐
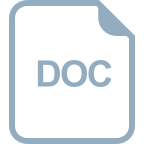
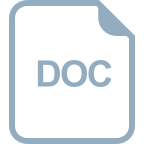
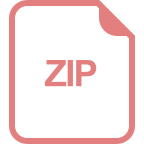
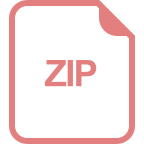
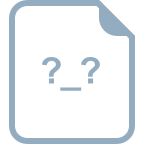
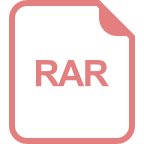











