GAM attention代码
时间: 2024-01-14 16:03:20 浏览: 123
以下是一个使用GAM(Global Attention Module)的示例代码:
```python
import torch
import torch.nn as nn
class GAM(nn.Module):
def __init__(self, in_channels):
super(GAM, self).__init__()
self.global_avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Sequential(
nn.Linear(in_channels, in_channels // 16),
nn.ReLU(inplace=True),
nn.Linear(in_channels // 16, in_channels),
nn.Sigmoid()
)
def forward(self, x):
b, c, _, _ = x.size()
y = self.global_avg_pool(x).view(b, c)
y = self.fc(y).view(b, c, 1, 1)
return x * y
# 使用GAM的示例网络
class MyNet(nn.Module):
def __init__(self):
super(MyNet, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.gam = GAM(64)
self.conv2 = nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1)
self.fc = nn.Linear(64, 10)
def forward(self, x):
x = self.conv1(x)
x = self.gam(x)
x = self.conv2(x)
x = nn.functional.avg_pool2d(x, 8)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
# 创建网络实例
net = MyNet()
```
这段代码定义了一个包含GAM的网络模型。GAM是一个全局注意力模块,用于增强网络对重要特征的关注。在示例中,我们定义了一个GAM类,它包含一个全局平均池化层和几个全连接层,用于计算特征图的注意力权重。然后,我们定义了一个包含GAM的网络模型MyNet,它使用GAM来增强特征图。最后,我们创建了一个网络实例net。
阅读全文
相关推荐
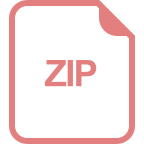

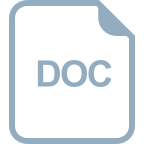
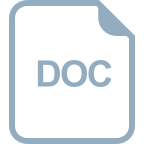





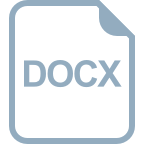
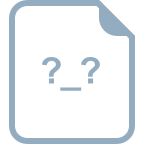
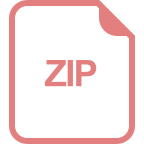