链表逆置pta
时间: 2023-07-10 20:20:12 浏览: 196
链表逆置是一个经典的算法问题,需要对链表的指针进行操作。你可以使用三个指针prev、cur、next,分别表示当前节点的前一个节点、当前节点和当前节点的后一个节点。具体步骤如下:
1. 初始化prev为NULL,cur为链表头结点。
2. 遍历链表,每次将cur指向的节点的next指针指向prev,然后将prev、cur、next三个指针依次向后移动一个节点,直到cur指向NULL为止。
3. 最后将链表的头结点指向prev,完成链表逆置。
以下是C++实现代码:
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* reverseList(ListNode* head) {
ListNode* prev = NULL;
ListNode* cur = head;
while (cur != NULL) {
ListNode* next = cur->next;
cur->next = prev;
prev = cur;
cur = next;
}
return prev;
}
int main() {
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
ListNode* new_head = reverseList(head);
while (new_head != NULL) {
cout << new_head->val << " ";
new_head = new_head->next;
}
cout << endl;
return 0;
}
```
输出结果为:4 3 2 1。
阅读全文
相关推荐
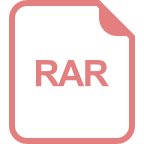

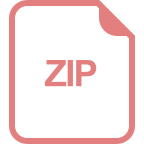
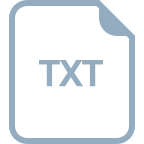
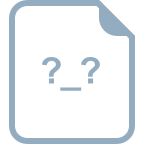
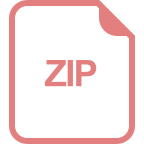
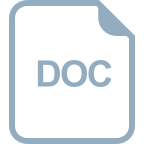
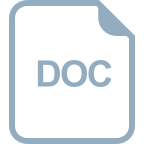
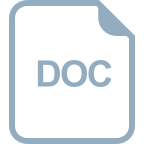
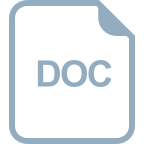
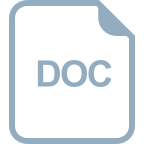

