android studio任务要求 1.创建一个界面 2.在界面中,使用按钮、开关按钮、进度条对话框,及其他必要组件 3.通过开关按钮,实现同一个文本框显示不同内容。 4.通过进度条对话框,显示下载进度。进度达到100%后,关闭对话框,并在界面中使用一个文本框显示下载完成。代码
时间: 2023-06-16 20:04:48 浏览: 60
下面是一个简单的示例代码,可以实现以上要求:
MainActivity.java
```java
import android.app.ProgressDialog;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ProgressBar;
import android.widget.Switch;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private EditText editText;
private TextView textView;
private Switch switchButton;
private Button downloadButton;
private ProgressBar progressBar;
private ProgressDialog progressDialog;
private int progress;
private Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
if (msg.what == 1) {
progressDialog.setProgress(progress);
if (progress == 100) {
progressDialog.dismiss();
textView.setText("下载完成");
}
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.edit_text);
textView = findViewById(R.id.text_view);
switchButton = findViewById(R.id.switch_button);
downloadButton = findViewById(R.id.download_button);
progressBar = findViewById(R.id.progress_bar);
downloadButton.setOnClickListener(this);
progressDialog = new ProgressDialog(MainActivity.this);
progressDialog.setTitle("下载中");
progressDialog.setMessage("请稍后...");
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.download_button:
download();
break;
}
}
private void download() {
progressDialog.show();
progress = 0;
new Thread(new Runnable() {
@Override
public void run() {
while (progress < 100) {
try {
Thread.sleep(100);
progress++;
handler.sendEmptyMessage(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
public void onSwitchClicked(View view) {
boolean checked = ((Switch) view).isChecked();
if (checked) {
editText.setText("开关已打开");
} else {
editText.setText("开关已关闭");
}
}
}
```
activity_main.xml
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/layout_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:ems="10"
android:hint="请输入内容"
android:inputType="textPersonName"
android:textAlignment="center"
android:textSize="24sp"
android:textStyle="bold" />
<Switch
android:id="@+id/switch_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/edit_text"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:text="开关按钮"
android:onClick="onSwitchClicked" />
<Button
android:id="@+id/download_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/switch_button"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:text="下载" />
<ProgressBar
android:id="@+id/progress_bar"
style="?android:attr/progressBarStyleHorizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/download_button"
android:layout_marginTop="16dp"
android:progress="0"
android:max="100"
android:visibility="visible" />
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/progress_bar"
android:layout_marginTop="16dp"
android:textAlignment="center"
android:textSize="24sp"
android:textStyle="bold" />
</RelativeLayout>
```
这个示例代码中,界面中包含一个文本框、一个开关按钮、一个下载按钮、一个进度条和一个文本框。
点击下载按钮后,会在界面中弹出一个进度条对话框,模拟下载过程,下载完成后会在文本框中显示“下载完成”。
点击开关按钮后,会在文本框中显示不同的内容,内容根据开关状态而定。
相关推荐
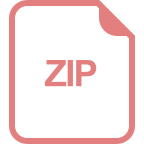
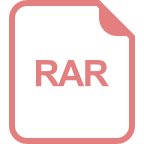
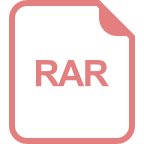















