用c sharp 编写一个贪吃蛇游戏
时间: 2023-06-12 11:06:42 浏览: 73
好的,以下是一个简单的贪吃蛇游戏的代码,使用 C# 编写:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SnakeGame
{
class Program
{
static void Main(string[] args)
{
// 设置游戏区域大小
int width = 20;
int height = 10;
// 初始化蛇的位置和方向
List<Point> snake = new List<Point>();
snake.Add(new Point(2, 0));
snake.Add(new Point(1, 0));
snake.Add(new Point(0, 0));
Direction direction = Direction.Right;
// 随机生成食物的位置
Point food = new Point(new Random().Next(width), new Random().Next(height));
// 游戏主循环
while (true)
{
// 清空屏幕
Console.Clear();
// 绘制游戏区域和蛇和食物
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
if (snake.Any(p => p.X == x && p.Y == y))
{
Console.Write("*");
}
else if (food.X == x && food.Y == y)
{
Console.Write("o");
}
else
{
Console.Write(".");
}
}
Console.WriteLine();
}
// 移动蛇的头部
Point head = snake[0];
switch (direction)
{
case Direction.Up:
head = new Point(head.X, head.Y - 1);
break;
case Direction.Right:
head = new Point(head.X + 1, head.Y);
break;
case Direction.Down:
head = new Point(head.X, head.Y + 1);
break;
case Direction.Left:
head = new Point(head.X - 1, head.Y);
break;
}
snake.Insert(0, head);
// 判断蛇是否吃到了食物
if (head.X == food.X && head.Y == food.Y)
{
// 生成新的食物
food = new Point(new Random().Next(width), new Random().Next(height));
}
else
{
// 移除蛇的尾部
snake.RemoveAt(snake.Count - 1);
}
// 判断游戏是否结束
if (head.X < 0 || head.X >= width || head.Y < 0 || head.Y >= height || snake.Any(p => p != head && p.X == head.X && p.Y == head.Y))
{
Console.WriteLine("Game over!");
Console.ReadKey();
return;
}
// 处理用户输入
if (Console.KeyAvailable)
{
ConsoleKeyInfo keyInfo = Console.ReadKey();
switch (keyInfo.Key)
{
case ConsoleKey.UpArrow:
direction = Direction.Up;
break;
case ConsoleKey.RightArrow:
direction = Direction.Right;
break;
case ConsoleKey.DownArrow:
direction = Direction.Down;
break;
case ConsoleKey.LeftArrow:
direction = Direction.Left;
break;
}
}
// 等待一段时间,控制游戏速度
System.Threading.Thread.Sleep(100);
}
}
}
// 表示一个点的类
class Point
{
public int X { get; set; }
public int Y { get; set; }
public Point(int x, int y)
{
X = x;
Y = y;
}
}
// 表示蛇的移动方向的枚举
enum Direction
{
Up,
Right,
Down,
Left
}
}
```
此代码使用控制台作为游戏界面,使用 `List<Point>` 表示蛇的身体,使用 `Point` 类表示游戏区域中的一个点,使用 `Direction` 枚举表示蛇的移动方向,使用 `Console.ReadKey()` 获取用户输入。您可以运行此代码,通过方向键控制蛇的移动。
相关推荐
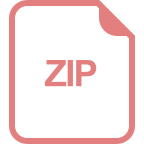
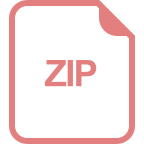
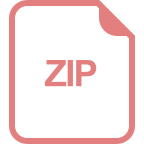
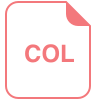
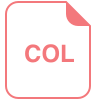
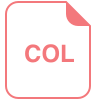
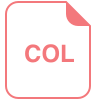
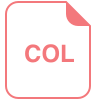









