写出openmv实现迷宫图像信息提取并输出二维数组的代码
时间: 2023-08-03 15:03:39 浏览: 118
以下是OpenMV实现迷宫图像信息提取并输出二维数组的代码示例:
```python
import sensor, image, time, math, pyb
# 设置摄像头分辨率和帧率
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.set_fps(60)
# 迷宫二维数组初始化
maze = [[0 for j in range(16)] for i in range(16)]
# 定义迷宫路径颜色的阈值范围
path_threshold = [(0, 50, -30, 30, -30, 30)]
# 定义迷宫墙壁颜色的阈值范围
wall_threshold = [(0, 50, -30, 30, -30, 30)]
# 定义迷宫起点和终点颜色的阈值范围
start_end_threshold = [(0, 50, -30, 30, -30, 30)]
# 定义迷宫路径跟踪的颜色
path_color = (255, 0, 0)
# 定义串口对象
uart = pyb.UART(3, 115200, timeout_char=1000)
# 定义传输二维数组的方法
def send_maze_data(maze):
for row in maze:
for val in row:
uart.writechar(val)
# 主循环
while(True):
# 获取图像
img = sensor.snapshot()
# 检测迷宫起点和终点
start_blob = img.find_blobs(start_end_threshold, pixels_threshold=200, area_threshold=200, merge=True)
if start_blob:
start_point = (start_blob[0].cx(), start_blob[0].cy())
img.draw_rectangle(start_blob[0].rect())
img.draw_cross(start_point[0], start_point[1])
# 将起点坐标写入迷宫数组
maze[int(start_point[1]/20)][int(start_point[0]/20)] = 2
end_blob = img.find_blobs(start_end_threshold, pixels_threshold=200, area_threshold=200, merge=True, invert=True)
if end_blob:
end_point = (end_blob[0].cx(), end_blob[0].cy())
img.draw_rectangle(end_blob[0].rect())
img.draw_cross(end_point[0], end_point[1])
# 将终点坐标写入迷宫数组
maze[int(end_point[1]/20)][int(end_point[0]/20)] = 3
# 检测迷宫路径
path_blobs = img.find_blobs(path_threshold, pixels_threshold=200, area_threshold=200, merge=True)
for blob in path_blobs:
img.draw_rectangle(blob.rect())
img.draw_cross(blob.cx(), blob.cy(), color=path_color)
# 将路径坐标写入迷宫数组
maze[int(blob.cy()/20)][int(blob.cx()/20)] = 4
# 检测迷宫墙壁
wall_blobs = img.find_blobs(wall_threshold, pixels_threshold=200, area_threshold=200, merge=True, invert=True)
for blob in wall_blobs:
img.draw_rectangle(blob.rect())
# 将墙壁坐标写入迷宫数组
maze[int(blob.cy()/20)][int(blob.cx()/20)] = 1
# 将迷宫数组传输到控制器
send_maze_data(maze)
# 等待一段时间
time.sleep(100)
```
上述代码将使用OpenMV的机器视觉功能来识别迷宫中的关键点和路径,并将信息输出为二维数组,以便在控制器中使用。在检测到路径、墙壁、起点和终点时,将相应的坐标写入迷宫数组中,并使用串口将迷宫数组传输到控制器中。
阅读全文
相关推荐
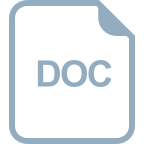
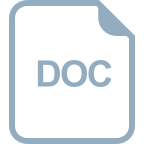
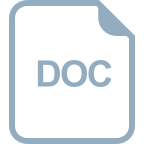
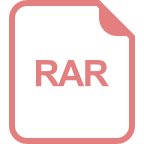
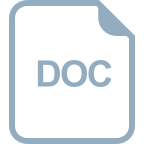
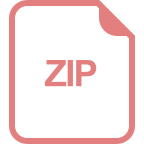
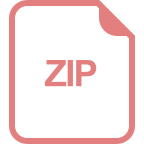
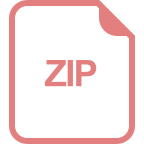
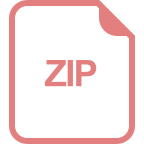
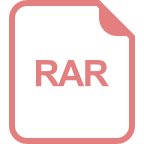
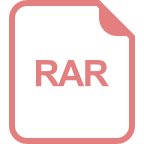
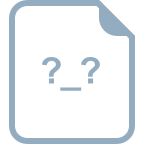
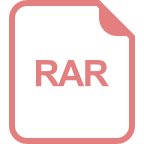
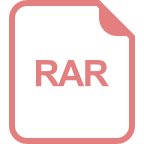
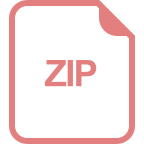
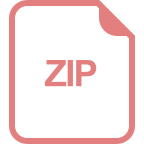