java excel转pdf 要求不能引用iText库
时间: 2024-05-05 10:20:01 浏览: 11
如果不使用iText库,你可以考虑使用Apache POI和Apache PDFBox这两个库来实现Excel转PDF的功能。具体步骤如下:
1.使用Apache POI读取Excel文件的内容,将内容渲染到PDF页面上。
2.使用Apache PDFBox创建PDF文档并将页面内容写入其中,生成PDF文件。
下面是一个Java示例代码,可以将Excel文件转换为PDF文件:
```
import java.io.*;
import org.apache.poi.hssf.usermodel.*;
import org.apache.poi.hssf.util.*;
import org.apache.pdfbox.pdmodel.*;
import org.apache.pdfbox.pdmodel.font.*;
import org.apache.pdfbox.pdmodel.graphics.image.*;
public class ExcelToPdfConverter {
public static void main(String[] args) throws Exception {
// Load Excel file
HSSFWorkbook workbook = new HSSFWorkbook(new FileInputStream("input.xls"));
// Load PDF document
PDDocument document = new PDDocument();
// Create a new PDF page
PDPage page = new PDPage(PDRectangle.A4);
document.addPage(page);
// Create a new content stream for the PDF page
PDPageContentStream contentStream = new PDPageContentStream(document, page);
// Set font and font size
PDFont font = PDType1Font.TIMES_ROMAN;
int fontSize = 12;
// Set table parameters
float margin = 72;
float tableWidth = page.getMediaBox().getWidth() - 2 * margin;
float yStartNewPage = page.getMediaBox().getHeight() - margin;
float yStart = yStartNewPage;
float bottomMargin = 70;
float yPosition = 650;
int rowsPerPage = 40;
// Create a table from the Excel file
HSSFSheet sheet = workbook.getSheetAt(0);
int rowCount = sheet.getPhysicalNumberOfRows();
int columnCount = sheet.getRow(0).getPhysicalNumberOfCells();
float[] columnWidths = new float[columnCount];
for (int i = 0; i < columnCount; i++) {
columnWidths[i] = tableWidth / columnCount;
}
// Draw table header
drawTableHeader(contentStream, yPosition, yStart, margin, tableWidth, font, fontSize, columnWidths, sheet);
// Draw table rows
int startRow = 1;
int endRow = Math.min(startRow + rowsPerPage, rowCount);
while (startRow < rowCount) {
drawTableRows(contentStream, yPosition, yStart, margin, tableWidth, font, fontSize, columnWidths, sheet, startRow, endRow);
endRow = Math.min(endRow + rowsPerPage, rowCount);
drawFooter(contentStream, bottomMargin, margin, tableWidth, font, fontSize);
document.addPage(page);
contentStream.close();
page = new PDPage(PDRectangle.A4);
document.addPage(page);
contentStream = new PDPageContentStream(document, page);
yStart = yStartNewPage;
startRow = endRow;
}
// Close the content stream
contentStream.close();
// Save the PDF document
document.save("output.pdf");
// Close the PDF document
document.close();
}
private static void drawTableHeader(PDPageContentStream contentStream, float yPosition, float yStart, float margin, float tableWidth, PDFont font, int fontSize, float[] columnWidths, HSSFSheet sheet) throws IOException {
contentStream.setFont(font, fontSize);
float currentPosition = yPosition;
for (int i = 0; i < columnWidths.length; i++) {
String columnHeader = sheet.getRow(0).getCell(i).getStringCellValue();
float columnWidth = columnWidths[i];
contentStream.beginText();
contentStream.moveTextPositionByAmount(margin + currentPosition, yStart);
contentStream.drawString(columnHeader);
contentStream.endText();
currentPosition += columnWidth;
}
}
private static void drawTableRows(PDPageContentStream contentStream, float yPosition, float yStart, float margin, float tableWidth, PDFont font, int fontSize, float[] columnWidths, HSSFSheet sheet, int startRow, int endRow) throws IOException {
contentStream.setFont(font, fontSize);
float currentPosition = yPosition;
for (int i = startRow; i < endRow; i++) {
float rowHeight = 0;
HSSFRow row = sheet.getRow(i);
for (int j = 0; j < columnWidths.length; j++) {
HSSFCell cell = row.getCell(j);
String cellValue = "";
if (cell != null) {
switch (cell.getCellType()) {
case HSSFCell.CELL_TYPE_BOOLEAN:
cellValue = Boolean.toString(cell.getBooleanCellValue());
break;
case HSSFCell.CELL_TYPE_NUMERIC:
cellValue = Double.toString(cell.getNumericCellValue());
break;
case HSSFCell.CELL_TYPE_STRING:
cellValue = cell.getStringCellValue();
break;
}
}
float columnWidth = columnWidths[j];
contentStream.beginText();
contentStream.moveTextPositionByAmount(margin + currentPosition, yStart);
contentStream.drawString(cellValue);
contentStream.endText();
currentPosition += columnWidth;
rowHeight = Math.max(rowHeight, contentStream.getFont().getFontDescriptor().getFontBoundingBox().getHeight() / 1000 * fontSize);
}
yStart -= rowHeight;
currentPosition = yPosition;
}
}
private static void drawFooter(PDPageContentStream contentStream, float bottomMargin, float margin, float tableWidth, PDFont font, int fontSize) throws IOException {
contentStream.setFont(font, fontSize);
contentStream.beginText();
contentStream.moveTextPositionByAmount(margin, bottomMargin);
contentStream.drawString("Generated by ExcelToPdfConverter");
contentStream.endText();
}
}
```
该示例代码使用了Apache POI和Apache PDFBox库来将Excel文件转换为PDF文件。你可以根据自己的需要进行修改和调整。
相关推荐
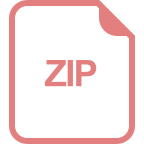






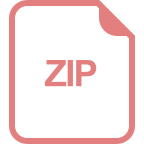
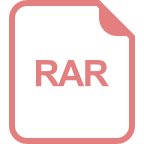
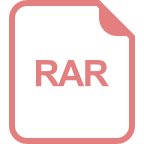
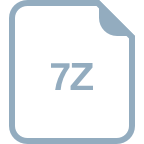
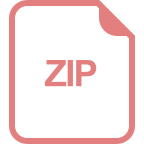
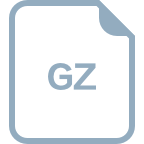
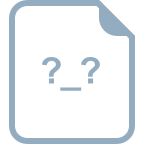