cad c# 利用drawjig 动态移动,旋转
时间: 2023-07-31 13:10:57 浏览: 302
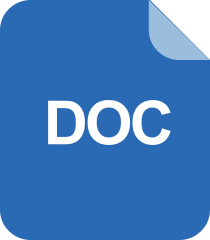
c#图像旋转
可以使用C#中的DrawJig类来实现动态移动和旋转。DrawJig类是用于绘制交互图形的基类,它提供了许多方法来实现交互式绘图。
以下是使用DrawJig类实现动态移动和旋转的示例代码:
```csharp
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Geometry;
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.GraphicsInterface;
namespace DynamicMoveRotate
{
public class MyDrawJig : DrawJig
{
private Point3d _basePoint;
private Vector3d _normal;
private double _angle;
private bool _move;
public MyDrawJig(Point3d basePoint, Vector3d normal)
{
_basePoint = basePoint;
_normal = normal;
_angle = 0.0;
_move = true;
}
protected override SamplerStatus Sampler(JigPrompts prompts)
{
if (_move)
{
JigPromptPointOptions jppo = new JigPromptPointOptions("\n指定移动点: ");
PromptPointResult ppr = prompts.AcquirePoint(jppo);
if (ppr.Status == PromptStatus.Cancel)
return SamplerStatus.Cancel;
if (_basePoint.DistanceTo(ppr.Value) > Tolerance.Global.EqualPoint)
{
_move = false;
_angle = _normal.GetAngleTo(ppr.Value - _basePoint);
}
}
else
{
JigPromptAngleOptions jpa = new JigPromptAngleOptions("\n指定旋转角度: ");
jpa.BasePoint = _basePoint;
jpa.UseBasePoint = true;
PromptDoubleResult pdr = prompts.AcquireAngle(jpa);
if (pdr.Status == PromptStatus.Cancel)
return SamplerStatus.Cancel;
_angle = pdr.Value;
}
return SamplerStatus.OK;
}
protected override bool WorldDraw(WorldDraw draw)
{
Vector3d axis = _normal.GetPerpendicularVector();
Matrix3d mat = Matrix3d.Rotation(_angle, axis, _basePoint);
draw.Geometry.Draw(new Line(_basePoint, _basePoint.TransformBy(mat)));
return true;
}
public ResultBuffer Run()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
PromptEntityOptions peo = new PromptEntityOptions("\n选择实体: ");
peo.SetRejectMessage("\n只能选择直线或圆弧!");
peo.AddAllowedClass(typeof(Line), true);
peo.AddAllowedClass(typeof(Circle), true);
PromptEntityResult per = ed.GetEntity(peo);
if (per.Status != PromptStatus.OK)
return null;
using (Transaction tr = doc.TransactionManager.StartTransaction())
{
Entity ent = tr.GetObject(per.ObjectId, OpenMode.ForWrite) as Entity;
if (ent == null)
return null;
_basePoint = ent.GeometricExtents.MinPoint;
_normal = ent.GetPlane().Normal;
MyDrawJig jig = new MyDrawJig(_basePoint, _normal);
PromptResult pr = ed.Drag(jig);
if (pr.Status != PromptStatus.OK)
return null;
Matrix3d mat = Matrix3d.Rotation(jig.Angle, _normal, _basePoint);
ent.TransformBy(mat);
tr.Commit();
}
return new ResultBuffer(new TypedValue[] { new TypedValue((int)DxfCode.Start, per.ObjectId) });
}
}
public class Commands
{
[CommandMethod("DYNMOVE")]
public void DynamicMove()
{
MyDrawJig jig = new MyDrawJig(Point3d.Origin, Vector3d.ZAxis);
jig.Run();
}
}
}
```
在这个示例中,我们创建了一个名为MyDrawJig的派生类来实现DrawJig。在Sampler方法中,我们首先采集移动点,然后在旋转点上采集旋转角度。在WorldDraw方法中,我们绘制了一个直线来表示移动和旋转的效果。最后,在Run方法中,我们选择实体,然后调用Drag方法来启动交互式绘图操作。
要使用此命令,请打开AutoCAD并输入"DYNMOVE"命令。然后,选择要移动和旋转的实体,按照提示来指定移动点和旋转角度即可。
阅读全文
相关推荐
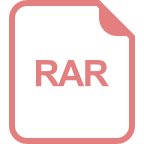
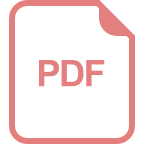
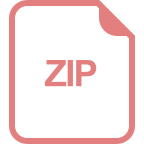
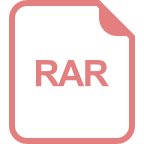
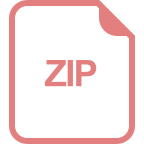
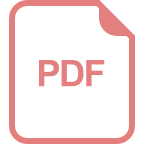
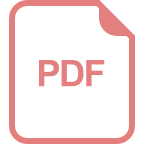
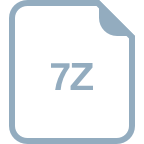
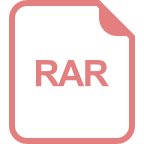
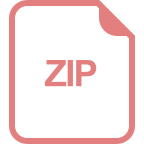
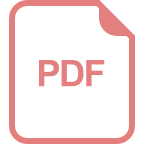
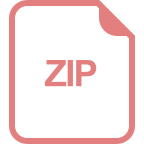
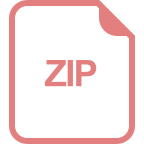