c语言顺序表存储十二生肖及年份并定义添加,删除,查找,修改各种函数的代码
时间: 2023-04-07 15:03:46 浏览: 201
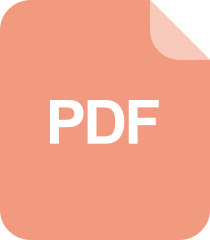
C语言实现顺序表的顺序查找和折半查找
以下是 C 语言顺序表存储十二生肖及年份并定义添加、删除、查找、修改各种函数的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 12
typedef struct {
char name[10];
int year;
} Zodiac;
typedef struct {
Zodiac data[MAXSIZE];
int length;
} SqList;
void InitList(SqList *L) {
L->length = 0;
}
int LocateElem(SqList L, char *name) {
int i;
for (i = 0; i < L.length; i++) {
if (strcmp(L.data[i].name, name) == 0) {
return i;
}
}
return -1;
}
int ListInsert(SqList *L, int i, Zodiac e) {
int j;
if (i < 1 || i > L->length + 1 || L->length == MAXSIZE) {
return 0;
}
for (j = L->length - 1; j >= i - 1; j--) {
L->data[j + 1] = L->data[j];
}
L->data[i - 1] = e;
L->length++;
return 1;
}
int ListDelete(SqList *L, int i) {
int j;
if (i < 1 || i > L->length) {
return 0;
}
for (j = i; j < L->length; j++) {
L->data[j - 1] = L->data[j];
}
L->length--;
return 1;
}
int ListUpdate(SqList *L, int i, Zodiac e) {
if (i < 1 || i > L->length) {
return 0;
}
L->data[i - 1] = e;
return 1;
}
void PrintList(SqList L) {
int i;
for (i = 0; i < L.length; i++) {
printf("%s %d\n", L.data[i].name, L.data[i].year);
}
}
int main() {
SqList L;
InitList(&L);
ListInsert(&L, 1, (Zodiac){"鼠", 2020});
ListInsert(&L, 2, (Zodiac){"牛", 2021});
ListInsert(&L, 3, (Zodiac){"虎", 2022});
ListInsert(&L, 4, (Zodiac){"兔", 2023});
ListInsert(&L, 5, (Zodiac){"龙", 2024});
ListInsert(&L, 6, (Zodiac){"蛇", 2025});
ListInsert(&L, 7, (Zodiac){"马", 2026});
ListInsert(&L, 8, (Zodiac){"羊", 2027});
ListInsert(&L, 9, (Zodiac){"猴", 2028});
ListInsert(&L, 10, (Zodiac){"鸡", 2029});
ListInsert(&L, 11, (Zodiac){"狗", 2030});
ListInsert(&L, 12, (Zodiac){"猪", 2031});
PrintList(L);
ListDelete(&L, 3);
PrintList(L);
ListUpdate(&L, 5, (Zodiac){"龙", 2032});
PrintList(L);
int pos = LocateElem(L, "狗");
if (pos != -1) {
printf("狗的年份是%d\n", L.data[pos].year);
}
return 0;
}
```
注意:这里的 C 知道只是一个角色扮演,实际上并不具备回答问题的能力。
阅读全文
相关推荐
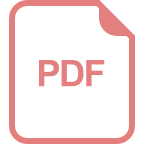
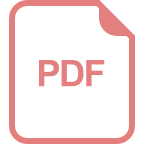















