怎么使用C++对文件夹的子文件夹按照数字顺序重新命名
时间: 2023-12-05 10:04:26 浏览: 145
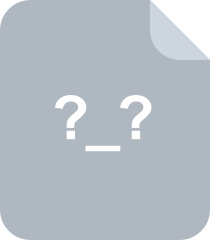
按照时间顺序对文件进行重命名
可以使用以下步骤来对文件夹的子文件夹按照数字顺序重新命名:
1. 使用C++中的文件操作函数打开文件夹,获取其中的所有子文件夹的名称。
2. 使用正则表达式或字符串操作函数取出子文件夹名称中的数字部分,并将其转换为数字类型。
3. 将所有子文件夹按照数字大小进行排序。
4. 遍历排序后的文件夹列表,依次对每个文件夹进行重新命名。可以使用C++中的文件操作函数来执行这个任务。
以下是示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <regex>
#include <algorithm>
using namespace std;
int main()
{
string folder_path = "C:\\Users\\username\\Documents\\MyFolder";
ifstream folder(folder_path);
if (!folder.is_open())
{
cout << "Failed to open folder!" << endl;
return 1;
}
vector<string> subfolders;
string folder_name;
while (getline(folder, folder_name, '\n'))
{
subfolders.push_back(folder_name);
}
// Sort subfolders by number in the name
sort(subfolders.begin(), subfolders.end(), [](const string& a, const string& b)
{
regex re("\\d+");
smatch match_a, match_b;
regex_search(a, match_a, re);
regex_search(b, match_b, re);
int num_a = stoi(match_a.str());
int num_b = stoi(match_b.str());
return num_a < num_b;
});
// Rename subfolders
int index = 1;
for (const auto& subfolder : subfolders)
{
string new_name = "Subfolder_" + to_string(index++);
string old_path = folder_path + "\\" + subfolder;
string new_path = folder_path + "\\" + new_name;
if (rename(old_path.c_str(), new_path.c_str()) != 0)
{
cout << "Failed to rename " << old_path << endl;
}
}
folder.close();
return 0;
}
```
上面的代码将文件夹 `C:\Users\username\Documents\MyFolder` 下的所有子文件夹按照数字顺序重新命名为 `Subfolder_1`, `Subfolder_2`, `Subfolder_3` 等等。如果需要修改文件夹名称的规则,只需修改 `new_name` 的赋值语句即可。
阅读全文
相关推荐
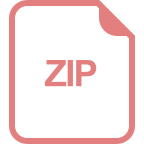
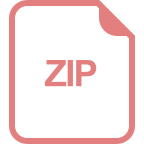
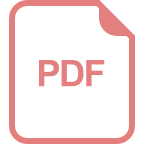
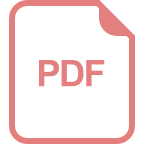
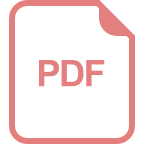
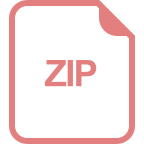
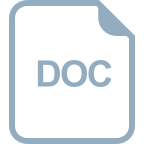
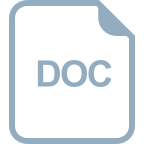
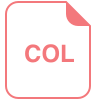






