用C语言设计一个航班管理系统,实现增删改查和排序的功能
时间: 2024-02-11 11:08:33 浏览: 21
好的,下面是一个基于结构体和指针的航班管理系统,它实现了增删改查和排序的功能。请注意,这只是一个示例,实际上需要更多的功能和完善的错误处理。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FLIGHTS 100
typedef struct {
char flight_number[10];
char origin[50];
char destination[50];
int departure_time;
} Flight;
Flight *flights[MAX_FLIGHTS];
int num_flights = 0;
void add_flight() {
if (num_flights >= MAX_FLIGHTS) {
printf("Too many flights in the system.\n");
return;
}
Flight *new_flight = (Flight *) malloc(sizeof(Flight));
if (new_flight == NULL) {
printf("Memory allocation failed.\n");
return;
}
printf("Enter flight number: ");
scanf("%s", new_flight->flight_number);
printf("Enter origin: ");
scanf("%s", new_flight->origin);
printf("Enter destination: ");
scanf("%s", new_flight->destination);
printf("Enter departure time: ");
scanf("%d", &new_flight->departure_time);
flights[num_flights++] = new_flight;
printf("Flight added successfully.\n");
}
void delete_flight() {
char flight_number[10];
int i, found = 0;
printf("Enter flight number to delete: ");
scanf("%s", flight_number);
for (i = 0; i < num_flights; i++) {
if (strcmp(flights[i]->flight_number, flight_number) == 0) {
found = 1;
break;
}
}
if (found) {
free(flights[i]);
for (; i < num_flights - 1; i++) {
flights[i] = flights[i + 1];
}
num_flights--;
printf("Flight deleted successfully.\n");
} else {
printf("Flight not found.\n");
}
}
void update_flight() {
char flight_number[10];
int i, found = 0;
printf("Enter flight number to update: ");
scanf("%s", flight_number);
for (i = 0; i < num_flights; i++) {
if (strcmp(flights[i]->flight_number, flight_number) == 0) {
found = 1;
break;
}
}
if (found) {
printf("Enter new origin: ");
scanf("%s", flights[i]->origin);
printf("Enter new destination: ");
scanf("%s", flights[i]->destination);
printf("Enter new departure time: ");
scanf("%d", &flights[i]->departure_time);
printf("Flight updated successfully.\n");
} else {
printf("Flight not found.\n");
}
}
void search_flight() {
char flight_number[10];
int i, found = 0;
printf("Enter flight number to search: ");
scanf("%s", flight_number);
for (i = 0; i < num_flights; i++) {
if (strcmp(flights[i]->flight_number, flight_number) == 0) {
found = 1;
printf("Flight %s from %s to %s departs at %d.\n",
flights[i]->flight_number, flights[i]->origin,
flights[i]->destination, flights[i]->departure_time);
break;
}
}
if (!found) {
printf("Flight not found.\n");
}
}
int compare_flights(const void *a, const void *b) {
Flight **flight_a = (Flight **) a;
Flight **flight_b = (Flight **) b;
return strcmp((*flight_a)->flight_number, (*flight_b)->flight_number);
}
void sort_flights() {
qsort(flights, num_flights, sizeof(Flight *), compare_flights);
printf("Flights sorted successfully.\n");
}
int main() {
int choice;
while (1) {
printf("\n1. Add flight\n");
printf("2. Delete flight\n");
printf("3. Update flight\n");
printf("4. Search flight\n");
printf("5. Sort flights\n");
printf("6. Quit\n");
printf("Enter choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_flight();
break;
case 2:
delete_flight();
break;
case 3:
update_flight();
break;
case 4:
search_flight();
break;
case 5:
sort_flights();
break;
case 6:
return 0;
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
这个代码和前面的示例很类似,但是有一些重要的改变。为了支持动态添加和删除航班,我们使用了指向 `Flight` 结构体的指针数组 `flights`,而不是以前的静态数组。当用户添加新的航班时,我们动态地分配一个新的 `Flight` 结构体,并将其地址存储在 `flights` 数组中。当用户删除航班时,我们释放相应的内存,并将后面的指针向前移动一位。
`update_flight()` 函数实现了更新航班的功能。它让用户输入要更新的航班号,并查找该航班是否存在。如果存在,它允许用户更新航班的起始地点、目的地和出发时间。
`compare_flights()` 函数是用来比较两个航班的航班号的辅助函数。它将两个 `Flight` 指针强制转换为 `Flight **`,并使用 `strcmp()` 函数比较航班号。
`sort_flights()` 函数实现了对航班按照航班号排序的功能。它使用 `qsort()` 函数,将 `flights` 数组中的航班指针按照航班号进行排序。
这个程序的控制流程和前面的示例类似,但是增加了更新和排序航班的选项。
相关推荐
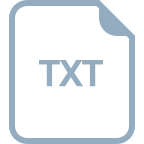
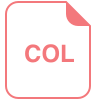
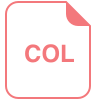
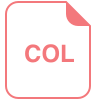
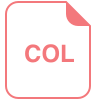
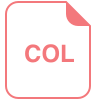









