停车场管理系统。分为管理员和用户。管理员实现管理停车场系统。如设置停车位、收费标准,车辆录入功能。 用户可以实现预约及停车。包含停车位查询,查询可用停车位,显示位置等,可被车牌号显示停车位置。 预约可预约的停车位 ,预约期也要收费的。收费,计算交费金额,收费C语言实现
时间: 2024-02-24 07:54:11 浏览: 37
好的,这是一个比较完整的停车场管理系统,需要实现管理员和用户两种角色的功能。
首先,我们需要定义一些数据结构来存储停车场信息,比如停车位数量、车辆信息等。可以使用结构体来存储这些信息,比如:
```c
// 停车位信息结构体
typedef struct {
int id; // 停车位编号
int status; // 停车位状态,0表示空闲,1表示占用
char license_plate[10]; // 车牌号
} ParkingSpot;
// 停车场信息结构体
typedef struct {
int total_spots; // 停车位总数
int available_spots; // 可用停车位数
float price_per_hour; // 每小时收费
ParkingSpot spots[MAX_SPOTS]; // 停车位数组
} ParkingLot;
// 车辆信息结构体
typedef struct {
char license_plate[10]; // 车牌号
time_t start_time; // 开始停车时间
time_t end_time; // 结束停车时间
float total_price; // 总费用
} Vehicle;
```
管理员可以通过设置停车位、收费标准、车辆录入等功能来管理停车场系统。这些功能可以通过命令行界面或者图形界面来实现。比如,设置停车位:
```c
void set_parking_spots(ParkingLot *lot, int total_spots) {
lot->total_spots = total_spots;
lot->available_spots = total_spots;
for (int i = 0; i < total_spots; i++) {
lot->spots[i].id = i + 1;
lot->spots[i].status = 0;
lot->spots[i].license_plate[0] = '\0';
}
}
```
用户可以实现预约及停车功能,包括停车位查询、预约停车位、停车等。用户可以通过命令行界面或者图形界面来实现。比如,查询可用停车位:
```c
int get_available_spots(ParkingLot *lot) {
return lot->available_spots;
}
```
预约停车位:
```c
int reserve_parking_spot(ParkingLot *lot, char *license_plate, int hours) {
if (lot->available_spots == 0) {
return -1; // 没有可用的停车位
}
// 查找第一个空闲的停车位
int spot_index = -1;
for (int i = 0; i < lot->total_spots; i++) {
if (lot->spots[i].status == 0) {
spot_index = i;
break;
}
}
if (spot_index == -1) {
return -1; // 没有可用的停车位
}
// 预约停车位
lot->spots[spot_index].status = 1;
strcpy(lot->spots[spot_index].license_plate, license_plate);
lot->available_spots--;
// 计算费用
float price = hours * lot->price_per_hour;
// 返回费用
return price;
}
```
停车:
```c
int park_vehicle(ParkingLot *lot, char *license_plate) {
// 查找车辆信息
Vehicle *vehicle = NULL;
for (int i = 0; i < MAX_VEHICLES; i++) {
if (vehicles[i].license_plate[0] != '\0' && strcmp(vehicles[i].license_plate, license_plate) == 0) {
vehicle = &vehicles[i];
break;
}
}
if (vehicle == NULL) {
return -1; // 没有该车辆信息
}
// 查找停车位
int spot_index = -1;
for (int i = 0; i < lot->total_spots; i++) {
if (lot->spots[i].status == 0) {
spot_index = i;
break;
}
}
if (spot_index == -1) {
return -1; // 没有可用的停车位
}
// 停车
lot->spots[spot_index].status = 1;
strcpy(lot->spots[spot_index].license_plate, license_plate);
lot->available_spots--;
vehicle->start_time = time(NULL);
return spot_index + 1; // 返回停车位编号
}
```
收费:
```c
float calculate_price(ParkingLot *lot, char *license_plate) {
// 查找车辆信息
Vehicle *vehicle = NULL;
for (int i = 0; i < MAX_VEHICLES; i++) {
if (vehicles[i].license_plate[0] != '\0' && strcmp(vehicles[i].license_plate, license_plate) == 0) {
vehicle = &vehicles[i];
break;
}
}
if (vehicle == NULL) {
return -1; // 没有该车辆信息
}
// 计算费用
time_t end_time = time(NULL);
float hours = difftime(end_time, vehicle->start_time) / 3600.0;
float price = hours * lot->price_per_hour;
vehicle->end_time = end_time;
vehicle->total_price = price;
// 释放停车位
for (int i = 0; i < lot->total_spots; i++) {
if (strcmp(lot->spots[i].license_plate, license_plate) == 0) {
lot->spots[i].status = 0;
lot->spots[i].license_plate[0] = '\0';
lot->available_spots++;
break;
}
}
return price;
}
```
以上是一个简单的停车场管理系统的实现,可以根据实际需要进行扩展和优化。
相关推荐
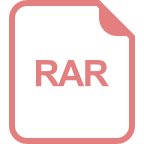














