springboot获取post参数
时间: 2023-04-24 09:06:03 浏览: 95
Spring Boot可以通过以下方式获取POST参数:
1. 使用@RequestBody注解将请求体转换为Java对象。
2. 使用@RequestParam注解获取请求参数。
3. 使用@PathVariable注解获取URL路径参数。
4. 使用HttpServletRequest对象获取请求参数。
5. 使用@ModelAttribute注解获取表单数据。
6. 使用@RequestHeader注解获取请求头信息。
具体使用方法可以参考Spring Boot官方文档或者相关教程。
相关问题
springboot 获取post取值
在Spring Boot中,您可以使用`@RequestParam`或`@RequestBody`注解来获取POST请求的参数。
1. 使用`@RequestParam`注解来获取POST请求参数值,示例下:
```java
("/example")
public String handlePostRequest(@RequestParam("param1") String param1, @RequestParam("param2") int param2) {
// 处理POST请求的逻辑
return "response";
}
```
在上述示例中,`@RequestParam`注解用于将请求中的参数值绑定到方法的参数上。您可以通过指定参数名来获取对应的参数值。
2. 使用`@RequestBody`注解来获取POST请求的请求体参数值,示例代码如下:
```java
@PostMapping("/example")
public String handlePostRequest(@RequestBody RequestObject request) {
// 处理POST请求的逻辑
return "response";
}
```
在上述示例中,`@RequestBody`注解用于将请求体的内容映射到`RequestObject`对象上。您可以通过定义一个与请求体内容匹配的POJO类来接收请求体参数值。
请根据您的具体需求选择合适的方式来获取POST请求的参数值。希望对您有所帮助!如果您有任何其他问题,请随时提问。
springboot 发送post请求 带参数
### 回答1:
可以使用 RestTemplate 发送带参数的 POST 请求,示例代码如下:
```java
RestTemplate restTemplate = new RestTemplate();
MultiValueMap<String, String> params = new LinkedMultiValueMap<>();
params.add("param1", "value1");
params.add("param2", "value2");
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
HttpEntity<MultiValueMap<String, String>> requestEntity = new HttpEntity<>(params, headers);
String url = "http://example.com/api";
String response = restTemplate.postForObject(url, requestEntity, String.class);
```
其中,params 是请求参数,headers 是请求头,requestEntity 是请求实体,url 是请求地址,response 是响应结果。
### 回答2:
使用Spring Boot发送带参数的POST请求,可以通过RestTemplate或者使用HttpClient来实现。以下是使用RestTemplate发送带参数的POST请求的示例代码:
```java
@RestController
public class MyController {
@Autowired
private RestTemplate restTemplate;
@PostMapping("/sendPost")
public String sendPostWithParams() {
String url = "http://example.com/api";
// 设置请求头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 设置请求参数
MultiValueMap<String, String> params = new LinkedMultiValueMap<>();
params.add("param1", "value1");
params.add("param2", "value2");
// 创建请求实体对象
HttpEntity<MultiValueMap<String, String>> requestEntity = new HttpEntity<>(params, headers);
// 发送POST请求并返回结果
ResponseEntity<String> responseEntity = restTemplate.postForEntity(url, requestEntity, String.class);
HttpStatus statusCode = responseEntity.getStatusCode();
String responseBody = responseEntity.getBody();
return "Status code: " + statusCode + "\n" + "Response body: " + responseBody;
}
}
```
在该示例中,首先通过`@Autowired`注解将`RestTemplate`自动注入到`MyController`中,然后在`sendPostWithParams`方法中构建请求头和请求参数。构建请求头时,我们设置了`Content-Type`为`application/json`,你也可以根据实际需求设置其他类型。构建请求参数时,我们使用了`MultiValueMap`来存储参数,可以根据需要添加更多参数。然后,将请求头和请求参数封装到`HttpEntity`对象中。最后使用`restTemplate`的`postForEntity`方法发送POST请求,并获取返回的状态码和响应体。最后将结果返回给客户端。
注意:在使用RestTemplate发送POST请求前,需要先添加RestTemplate的依赖,例如在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
</dependencies>
```
然后,你需要在Spring Boot应用的配置类中添加一个`@Bean`注解来创建`RestTemplate`的实例,例如:
```java
@Configuration
public class AppConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
```
以上就是使用Spring Boot发送带参数的POST请求的一个简单示例。
### 回答3:
在Spring Boot中发送带参数的POST请求,可以通过使用RestTemplate或者HttpClient来实现。
使用RestTemplate发送POST请求的示例代码如下:
```
import org.springframework.http.*;
import org.springframework.web.client.RestTemplate;
public class PostRequestExample {
public static void main(String[] args) {
// 创建RestTemplate对象
RestTemplate restTemplate = new RestTemplate();
// 构造请求体和请求头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 构造请求参数
MultiValueMap<String, Object> params = new LinkedMultiValueMap<>();
params.add("param1", "value1");
params.add("param2", "value2");
// 创建HttpEntity对象,设置请求体和请求头
HttpEntity<MultiValueMap<String, Object>> requestEntity = new HttpEntity<>(params, headers);
// 发送POST请求
ResponseEntity<String> response = restTemplate.exchange("URL", HttpMethod.POST, requestEntity, String.class);
// 获取响应结果
String responseBody = response.getBody();
System.out.println(responseBody);
}
}
```
上述代码中,我们通过RestTemplate发送了一个POST请求,并且设置了请求参数。其中,`param1`和`param2`是请求参数的键,对应的`value1`和`value2`是请求参数的值。
另外,还需要自定义请求头`Content-Type`为`application/json`,根据实际情况设置请求URL地址,然后使用RestTemplate的`exchange`方法发送POST请求。
当然,你也可以使用HttpClient来发送POST请求。使用HttpClient发送POST请求的示例代码如下:
```
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class PostRequestExample {
public static void main(String[] args) throws Exception {
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 创建HttpPost对象,并设置请求URL
HttpPost httpPost = new HttpPost("URL");
// 构造请求参数
StringEntity params = new StringEntity("{\"param1\":\"value1\",\"param2\":\"value2\"}");
// 设置请求头和请求体
httpPost.addHeader("Content-Type", "application/json");
httpPost.setEntity(params);
// 发送POST请求
CloseableHttpResponse response = httpClient.execute(httpPost);
// 获取响应结果
HttpEntity entity = response.getEntity();
String responseBody = EntityUtils.toString(entity);
System.out.println(responseBody);
// 关闭资源
response.close();
httpClient.close();
}
}
```
上述代码中,我们通过HttpClient发送了一个POST请求,并且设置了请求参数和请求头。其中,请求参数是一个JSON字符串,根据实际情况设置请求URL地址。通过设置请求头`Content-Type`为`application/json`,然后使用HttpClient的`execute`方法发送POST请求。
以上就是使用Spring Boot发送带参数的POST请求的示例代码,你可以根据实际情况进行调整和扩展。
阅读全文
相关推荐
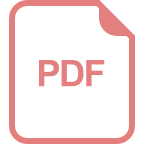
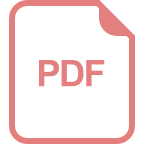
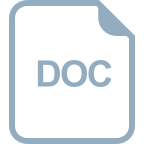
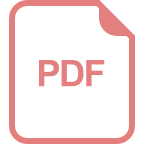
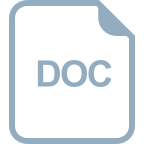










