用波兰表达式求值,用c++编程
时间: 2024-02-27 16:57:57 浏览: 16
好的,让我来帮你回答这个问题。
首先,波兰表达式是一种前缀表达式,其中运算符在操作数之前。例如,表达式 "2 + 3" 在波兰表示法中写作 "+ 2 3"。
要求解波兰表达式,可以使用一个栈来辅助计算。遍历表达式中的每个元素,如果遇到操作数,则将其压入栈中;如果遇到运算符,则从栈中弹出相应数量的操作数,进行计算,并将结果压入栈中。最终,栈中仅剩下一个元素,即为表达式的值。
下面是用 C++ 实现波兰表达式求值的代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int evaluate(string expr) {
stack<int> operands;
for (int i = expr.size() - 1; i >= 0; i--) {
if (isdigit(expr[i])) {
operands.push(expr[i] - '0');
} else if (isspace(expr[i])) {
// do nothing
} else {
int op1 = operands.top(); operands.pop();
int op2 = operands.top(); operands.pop();
switch (expr[i]) {
case '+': operands.push(op1 + op2); break;
case '-': operands.push(op1 - op2); break;
case '*': operands.push(op1 * op2); break;
case '/': operands.push(op1 / op2); break;
}
}
}
return operands.top();
}
int main() {
string expr = "+ * 2 3 4";
int result = evaluate(expr);
cout << "Result: " << result << endl;
return 0;
}
```
在上面的代码中,我们使用了一个 `stack<int>` 类型的栈来辅助计算。遍历表达式 `expr` 中的每个元素,如果遇到操作数,则将其转换为整数并压入栈中;如果遇到运算符,则从栈中弹出相应数量的操作数,进行计算,并将结果压入栈中。最终,栈中仅剩下一个元素,即为表达式的值。
相关推荐
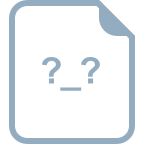














