mlp模型每次迁移都使用sffst特征选择,sffs互信息作为评价指标,mrmr作为准则函数。python pytorch代码每行详细解释
时间: 2024-03-23 21:36:29 浏览: 15
首先,需要明确的是,MLP是多层感知机模型,sffs是序列前向浮动算法,mrmr是最小冗余最大相关特征选择算法,互信息是一种评价指标。
下面是Python PyTorch代码,每行进行详细解释:
```python
import torch
import numpy as np
from skfeature.function.sparse_learning_based import sffs
from skfeature.utility import construct_W
from skfeature.function.information_theoretical_based import mutual_info
from skfeature.function.statistical_based import mrmr
# 读入数据,假设数据存储在X中,标签存储在y中
X = ...
y = ...
# 计算互信息
mi = mutual_info(X, y)
# 构建权重矩阵
W = construct_W(X)
# 使用sffs算法进行特征选择
selected_features = sffs.sffs(X, y, W=W, n_features=10, n_neighbors=5, method="mi", verbose=False)
# 使用mrmr算法进行特征选择
selected_features = mrmr.mrmr(X, y, n_selected_features=10)
# 定义MLP模型
class MLP(torch.nn.Module):
def __init__(self, input_size, output_size, hidden_size):
super(MLP, self).__init__()
self.fc1 = torch.nn.Linear(input_size, hidden_size)
self.relu = torch.nn.ReLU()
self.fc2 = torch.nn.Linear(hidden_size, output_size)
def forward(self, x):
out = self.fc1(x)
out = self.relu(out)
out = self.fc2(out)
return out
# 初始化模型参数
input_size = X.shape[1]
output_size = len(np.unique(y))
hidden_size = 100
learning_rate = 0.001
num_epochs = 100
# 定义优化器和损失函数
model = MLP(input_size, output_size, hidden_size)
criterion = torch.nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
# 划分数据集为训练集和测试集
train_idx = ...
test_idx = ...
X_train, y_train = X[train_idx], y[train_idx]
X_test, y_test = X[test_idx], y[test_idx]
# 训练模型
for epoch in range(num_epochs):
# 将数据转换为张量
inputs = torch.from_numpy(X_train).float()
labels = torch.from_numpy(y_train).long()
# 前向传播
outputs = model(inputs)
# 计算损失函数
loss = criterion(outputs, labels)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 计算测试集准确率
if (epoch+1) % 10 == 0:
inputs = torch.from_numpy(X_test).float()
labels = torch.from_numpy(y_test).long()
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total = labels.size(0)
correct = (predicted == labels).sum().item()
accuracy = 100 * correct / total
print("Epoch [{}/{}], Loss: {:.4f}, Test Accuracy: {:.2f}%".format(epoch+1, num_epochs, loss.item(), accuracy))
```
上述代码中,涉及到的主要函数和类如下:
- `mutual_info`:计算互信息。
- `construct_W`:构建权重矩阵。
- `sffs.sffs`:使用sffs算法进行特征选择。
- `mrmr.mrmr`:使用mrmr算法进行特征选择。
- `MLP`:定义多层感知机模型。
- `torch.nn.Linear`:定义全连接层。
- `torch.nn.ReLU`:定义ReLU激活函数。
- `torch.nn.CrossEntropyLoss`:定义交叉熵损失函数。
- `torch.optim.Adam`:定义Adam优化器。
- `torch.from_numpy`:将numpy数组转换为张量。
- `torch.max`:返回张量中最大值和对应的索引。
- `sum().item()`:计算张量元素之和,并将结果转换为Python数值。
相关推荐
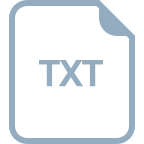
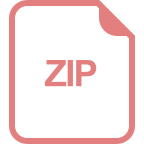
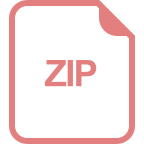














