implement the overlap-add algorithm through the matlab
时间: 2024-01-22 19:19:28 浏览: 100
Sure, here's an implementation of the overlap-add algorithm in MATLAB:
```matlab
function x = overlap_add(h, y, N)
% h: FIR filter impulse response
% y: input signal
% N: block size (must be power of 2)
M = length(h); % length of filter impulse response
L = N-M+1; % hop size
x = zeros(1, length(y)+M-1); % output signal
% FFT of filter impulse response
H = fft(h, N);
% process blocks of input signal
for i = 1:L:length(y)
% take a block of input signal
if i+L-1 <= length(y)
y_block = y(i:i+L-1);
else
y_block = [y(i:end) zeros(1, i+L-1-length(y))];
end
% zero-pad input block to length N
y_block = [y_block zeros(1, N-L)];
% FFT of input block
Y = fft(y_block);
% convolution in frequency domain
X = Y .* H;
% inverse FFT to get time-domain output
x_block = ifft(X);
% add output block to output signal
x(i:i+N-1) = x(i:i+N-1) + x_block;
end
% remove padding from start and end of output signal
x = x(M:end-M+1);
```
To use this function, you can call it with the filter impulse response `h`, the input signal `y`, and the block size `N`. The output signal `x` will be returned.
Note that the block size `N` must be a power of 2 for efficient FFT computation. Also, the length of the filter impulse response `h` must be less than or equal to the block size `N`. If it's longer, you can zero-pad it to length `N`.
阅读全文
相关推荐
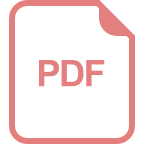





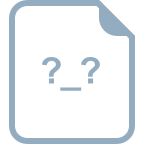









