如何使用arduino连接onenet
时间: 2023-08-14 08:02:12 浏览: 182
要使用Arduino连接OneNet,需要进行以下步骤:
1. 创建OneNet账户并创建设备:在OneNet平台上创建一个账户并创建一个设备来接收来自Arduino的数据。
2. 下载并安装OneNet库:在Arduino IDE中安装OneNet库,这个库可以帮助我们将数据发送到OneNet平台。
3. 编写Arduino代码:在Arduino IDE中编写代码,包括WiFi连接代码和OneNet数据上传代码。
4. 测试连接:将Arduino连接到电脑,上传代码并测试连接,确保数据能够成功发送到OneNet平台。
以下是一个简单的示例代码,可以用于连接Arduino Uno和OneNet平台:
```
#include <OneNet.h>
#include <SPI.h>
#include <WiFi.h>
char ssid[] = "your_SSID"; // your network SSID (name)
char password[] = "your_PASSWORD"; // your network password (use for WPA, or use as key for WEP)
int status = WL_IDLE_STATUS; // the Wifi radio's status
char* device_id = "your_DEVICE_ID"; // 设备ID
char* api_key = "your_API_KEY"; // API Key
OneNet onenet(device_id, api_key);
void setup() {
// initialize serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// check for the presence of the shield:
if (WiFi.status() == WL_NO_SHIELD) {
Serial.println("WiFi shield not present");
// don't continue:
while (true);
}
// attempt to connect to Wifi network:
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network. Change this line if using open or WEP network:
status = WiFi.begin(ssid, password);
// wait 5 seconds for connection:
delay(5000);
}
// you're connected now, so print out the data:
Serial.print("You're connected to the network");
}
void loop() {
// read sensor data and store in variables
int sensorValue = analogRead(A0);
float voltage = sensorValue * (5.0 / 1023.0);
// create JSON object with sensor data
JsonObject data = JsonObject();
data.addFloat("voltage", voltage);
// send data to OneNet
bool success = onenet.add_data(data);
if (success) {
Serial.println("Data sent successfully");
} else {
Serial.println("Data failed to send");
}
// wait for 5 seconds before sending the next data point
delay(5000);
}
```
请注意,这里的 `your_SSID` 和 `your_PASSWORD` 需要根据你的WiFi网络进行替换,`your_DEVICE_ID` 和 `your_API_KEY` 需要根据你在OneNet上创建的设备进行替换。
阅读全文
相关推荐
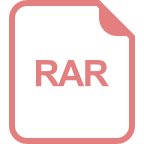
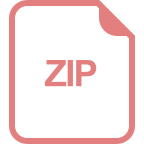
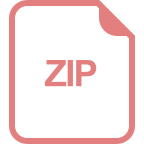















