检测灰度图中白色和黑色的分界线并拟合直线 c++
时间: 2024-04-23 09:23:45 浏览: 21
好的,以下是使用 C++ 实现检测灰度图中白色和黑色的分界线并拟合直线的代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取灰度图像
Mat img_gray = imread("gray_image.jpg", IMREAD_GRAYSCALE);
// 二值化图像
Mat img_binary;
threshold(img_gray, img_binary, 127, 255, THRESH_BINARY);
// Canny边缘检测
Mat img_edges;
Canny(img_binary, img_edges, 100, 200);
// 霍夫直线变换
vector<Vec2f> lines;
HoughLines(img_edges, lines, 1, CV_PI/180, 100);
// 选择斜率最接近水平或垂直的直线,并将其拟合为一条直线
float max_theta = 0;
float max_length = 0;
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0];
float theta = lines[i][1];
float length = abs(rho);
if (length > max_length && (abs(theta - CV_PI/2) < CV_PI/6 || abs(theta) < CV_PI/6))
{
max_length = length;
max_theta = theta;
}
}
Point2f pt1, pt2;
if (abs(max_theta - CV_PI/2) < CV_PI/6)
{
float line_y = cos(max_theta) / sin(max_theta);
float y_intercept = img_gray.cols / 2 - line_y * img_gray.rows / 2;
pt1 = Point2f(0, y_intercept);
pt2 = Point2f(img_gray.cols, line_y * img_gray.cols + y_intercept);
}
else
{
float line_x = sin(max_theta) / cos(max_theta);
float x_intercept = img_gray.rows / 2 - line_x * img_gray.cols / 2;
pt1 = Point2f(x_intercept, 0);
pt2 = Point2f(line_x * img_gray.rows + x_intercept, img_gray.rows);
}
// 在原始图像上绘制直线
Mat img_output = imread("gray_image.jpg", IMREAD_COLOR);
line(img_output, pt1, pt2, Scalar(0, 255, 0), 2);
// 显示结果
imshow("Output", img_output);
waitKey();
return 0;
}
```
这里使用了 OpenCV 库中的函数来实现图像的读取、二值化、Canny 边缘检测、霍夫直线变换和直线绘制等操作。希望这份代码能对您有所帮助!
相关推荐
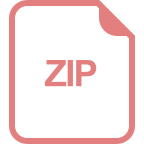














