java编程设计一个简单的学生信息管理系统。每个学生的信息包括学号、姓名、性别、班级和电话等。 实验要求: (1) 采用二叉排序树的结构创建学生的信息表。 ⑵ 能按照学号或姓名查找学生的信息。如果查找成功,则输出学生的所有信息,否则输入查找失败的提示信息。
时间: 2024-05-09 14:17:14 浏览: 38
您好!感谢您的问题,我将为您提供帮助。
针对您的问题,我可以提供如下的Java代码示例:
```java
import java.util.Scanner;
class Student {
public int id;
public String name;
public String gender;
public String className;
public String phone;
public Student(int id, String name, String gender, String className, String phone) {
this.id = id;
this.name = name;
this.gender = gender;
this.className = className;
this.phone = phone;
}
public String toString() {
return "学号:" + id + ",姓名:" + name + ",性别:" + gender + ",班级:" + className + ",电话:" + phone;
}
}
class StudentTreeNode {
public Student student;
public StudentTreeNode left;
public StudentTreeNode right;
public StudentTreeNode(Student student) {
this.student = student;
this.left = null;
this.right = null;
}
}
class StudentTree {
public StudentTreeNode root;
public StudentTree() {
this.root = null;
}
public void insert(Student student) {
if (this.root == null) {
this.root = new StudentTreeNode(student);
return;
}
StudentTreeNode currentNode = this.root;
while (true) {
if (student.id < currentNode.student.id) {
if (currentNode.left == null) {
currentNode.left = new StudentTreeNode(student);
return;
} else {
currentNode = currentNode.left;
}
} else {
if (student.id > currentNode.student.id) {
if (currentNode.right == null) {
currentNode.right = new StudentTreeNode(student);
return;
} else {
currentNode = currentNode.right;
}
} else {
return;
}
}
}
}
public StudentTreeNode find(int id) {
StudentTreeNode currentNode = this.root;
while (currentNode != null) {
if (id < currentNode.student.id) {
currentNode = currentNode.left;
} else {
if (id > currentNode.student.id) {
currentNode = currentNode.right;
} else {
return currentNode;
}
}
}
return null;
}
public StudentTreeNode find(String name) {
StudentTreeNode currentNode = this.root;
while (currentNode != null) {
if (name.compareTo(currentNode.student.name) < 0) {
currentNode = currentNode.left;
} else {
if (name.compareTo(currentNode.student.name) > 0) {
currentNode = currentNode.right;
} else {
return currentNode;
}
}
}
return null;
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
StudentTree studentTree = new StudentTree();
System.out.println("请输入学生信息,输入-1结束:");
while (true) {
System.out.print("学号:");
int id = scanner.nextInt();
if (id == -1) {
break;
}
System.out.print("姓名:");
String name = scanner.next();
System.out.print("性别:");
String gender = scanner.next();
System.out.print("班级:");
String className = scanner.next();
System.out.print("电话:");
String phone = scanner.next();
Student student = new Student(id, name, gender, className, phone);
studentTree.insert(student);
}
while (true) {
System.out.println("请选择查找方式(1-按学号查找,2-按姓名查找,0-退出):");
int choice = scanner.nextInt();
if (choice == 0) {
break;
}
if (choice == 1) {
System.out.print("请输入学号:");
int id = scanner.nextInt();
StudentTreeNode studentNode = studentTree.find(id);
if (studentNode == null) {
System.out.println("查找失败!");
} else {
System.out.println(studentNode.student);
}
}
if (choice == 2) {
System.out.print("请输入姓名:");
String name = scanner.next();
StudentTreeNode studentNode = studentTree.find(name);
if (studentNode == null) {
System.out.println("查找失败!");
} else {
System.out.println(studentNode.student);
}
}
}
}
}
```
代码中定义了一个 `Student` 类,表示学生信息,包括学号、姓名、性别、班级和电话等。还定义了一个二叉排序树 `StudentTree`,用于存储学生信息,并支持按学号或姓名查找学生信息。在 `main()` 函数中,先读入一些学生信息,然后循环提供查找功能,输入 1 表示按学号查找,输入 2 表示按姓名查找,输入 0 表示退出。每次查找后,输出学生信息或查找失败的提示信息。
希望这些代码能够帮助到您,如果您有其他问题或需要进一步帮助,请随时告诉我。
阅读全文
相关推荐




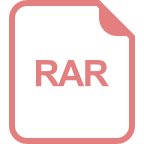

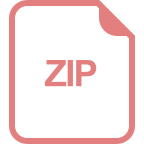

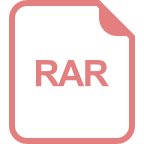
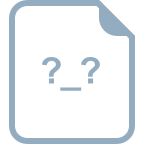
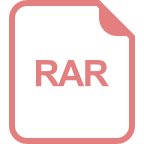

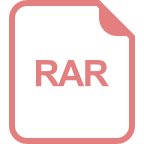




