springboot启动定时任务
时间: 2023-08-31 16:27:11 浏览: 61
### 回答1:
SpringBoot 可以使用 @Scheduled 注解来实现定时任务。该注解可以标注在方法上,指示该方法是一个定时任务。例如:
```
@Scheduled(fixedRate = 1000)
public void reportCurrentTime() {
System.out.println("当前时间:" + dateFormat.format(new Date()));
}
```
其中,`fixedRate` 属性指定了任务的执行频率,单位是毫秒。该示例代码将会每隔 1 秒打印一次当前时间。
如果要启动定时任务,可以在启动类中添加如下代码:
```
@EnableScheduling
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
其中,`@EnableScheduling` 注解用来启用定时任务功能,`@SpringBootApplication` 注解用来启动 SpringBoot 应用。
### 回答2:
在Spring Boot中启动定时任务非常简单。首先,需要在应用程序的主类上添加注解@EnableScheduling,该注解表明应用程序将使用Spring的调度功能。然后,在需要定时执行的方法上添加注解@Scheduled,指定执行的时间间隔或固定的执行时间。
以下是一个简单的示例,演示如何在Spring Boot中启动定时任务:
首先,确保在pom.xml文件中添加了spring-boot-starter-web依赖,以支持Spring Boot的Web功能。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
然后,在应用程序的主类上添加注解@EnableScheduling:
```java
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
接下来,在需要定时执行的方法上添加注解@Scheduled,并指定执行的时间间隔或固定的执行时间。例如,以下代码将每5秒执行一次:
```java
@Component
public class MyTask {
@Scheduled(fixedRate = 5000)
public void executeTask() {
System.out.println("定时任务执行中...");
}
}
```
在上述示例中,MyTask类使用@Component注解将其标记为Spring Bean。在Spring Boot中,所有带有@Component注解的类都会自动被扫描并注册为Bean。因此,Spring会自动创建一个名为myTask的Bean,并根据@Scheduled注解的配置定期执行executeTask()方法。
通过以上步骤,我们可以实现在Spring Boot中启动定时任务。当应用程序启动后,定时任务将按照指定的时间间隔或固定时间开始执行。
### 回答3:
在Spring Boot中启动定时任务非常简单。首先,我们需要在项目的依赖管理文件(通常是pom.xml)中添加Spring Boot定时任务的依赖。可以通过在 `<dependencies>` 标签中添加以下代码来添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
接下来,我们需要创建一个任务类,该类负责实现我们要执行的定时任务逻辑。我们可以使用`@Component`注解标记该类为Spring组件,以便我们可以在应用程序中使用它。
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyTask {
@Scheduled(cron = "0 0/5 * * * ?") // 每5分钟执行一次
public void executeTask() {
// 定时任务的逻辑代码写在这里
}
}
```
在上面的示例中,我们定义了一个使用cron表达式的定时任务,该任务将每5分钟执行一次。
最后,我们需要在Spring Boot应用程序的入口类上添加`@EnableScheduling`注解,以启用定时任务功能。
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
```
现在,当我们运行Spring Boot应用程序时,定时任务将按照我们定义的计划执行。
需要注意的是,Spring Boot使用了Spring框架的`@Scheduled`注解来实现定时任务的功能。除了cron表达式之外,我们还可以使用其他类型的表达式来定义不同时间间隔的定时任务。
相关推荐
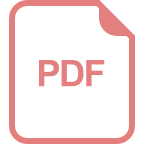
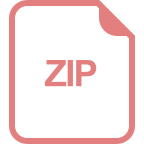














