dict_ = df['Medium for online class'].value_counts().to_dict() plt.figure(figsize=(12,12)) plt.pie(x=dict_.values(), startangle=0, explode=[0, 0.01, 0.05, 0.1, 0.2]) plt.legend(labels=dict_.keys(), loc='upper right',shadow=True, facecolor='lightyellow') plt.show()
时间: 2023-07-08 20:22:30 浏览: 130
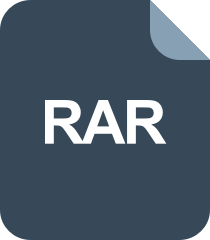
09_dict_key.rar_Word for Word_dictionary_grandfather84l
这是一个关于数据可视化的代码,使用了 pandas 和 matplotlib 库。首先,从一个名为 df 的数据帧中获取了一个名为 Medium for online class 的列,然后使用 value_counts() 方法计算该列中每个值的数量,将其转换为一个字典 dict_。接下来,创建一个大小为 12x12 的图形,使用 pie() 方法绘制一个饼图,其中 x 参数为 dict_ 的值,startangle 参数为 0,explode 参数指定了每个扇形离中心的距离,legend() 方法添加了标签和图例。最后,使用 show() 方法显示图形。
阅读全文
相关推荐
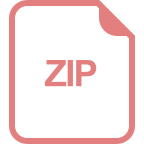
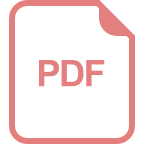








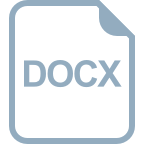
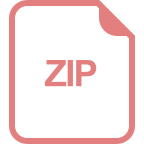





