#include <wx/wx.h> #include <vector> #include <fstream> #include <sstream> #include<string> std::vector<wxPoint> west_outline_pix_position{}; class MyFrame : public wxFrame { public: MyFrame() : wxFrame(nullptr, wxID_ANY, "File Example", wxDefaultPosition, wxSize(500, 500)) { // 读取txt文件中所有纬度、经度数据 readTxtFile("data.txt"); // 测试输出 for (const wxPoint& point : west_outline_pix_position) { wxLogMessage("Longitude: %f, Latitude: %f", point.x, point.y); } } void readTxtFile(std::string filename) { std::ifstream file(filename); if (!file.is_open()) { wxLogError("Failed to open file!"); return; } std::string line; //while (std::getline(file, line)) { // wxLogMessage("Read line: %s", line); // // 其他代码 //} while (std::getline(file, line)) { std::istringstream iss(line); std::string token; std::vector<double> values; while (std::getline(iss, token, ',')) { double value = std::stoi(token); values.push_back(value); // 将 value 存入向量中 } double longitude, latitude; if (values.size() < 2) { wxLogError("Failed to read data from file!"); return; } longitude = values[0]; latitude = values[1]; west_outline_pix_position.emplace_back(wxPoint(longitude, latitude)); } } }; class MyApp : public wxApp { public: bool OnInit() { MyFrame* frame = new MyFrame(); frame->Show(); return true; } }; wxIMPLEMENT_APP(MyApp);
时间: 2024-03-01 21:50:42 浏览: 20
您可以将代码中的 wxPoint 类型改为 wxRealPoint 类型,这样就可以输出 double 类型的值了。
修改后的代码如下:
```c++
#include <wx/wx.h>
#include <vector>
#include <fstream>
#include <sstream>
#include <string>
std::vector<wxRealPoint> west_outline_pix_position{};
class MyFrame : public wxFrame {
public:
MyFrame() : wxFrame(nullptr, wxID_ANY, "File Example", wxDefaultPosition, wxSize(500, 500)) {
// 读取txt文件中所有纬度、经度数据
readTxtFile("data.txt");
// 测试输出
for (const wxRealPoint& point : west_outline_pix_position) {
wxLogMessage("Longitude: %lf, Latitude: %lf", point.x, point.y);
}
}
void readTxtFile(std::string filename)
{
std::ifstream file(filename);
if (!file.is_open()) {
wxLogError("Failed to open file!");
return;
}
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
std::string token;
std::vector<double> values;
while (std::getline(iss, token, ',')) {
double value = std::stod(token); // 修改此处,使用 stod 函数将字符串转换为 double 类型
values.push_back(value);
}
double longitude, latitude;
if (values.size() < 2) {
wxLogError("Failed to read data from file!");
return;
}
longitude = values[0];
latitude = values[1];
west_outline_pix_position.emplace_back(wxRealPoint(longitude, latitude)); // 修改此处,使用 wxRealPoint 类型
}
}
};
class MyApp : public wxApp {
public:
bool OnInit() {
MyFrame* frame = new MyFrame();
frame->Show();
return true;
}
};
wxIMPLEMENT_APP(MyApp);
```
相关推荐
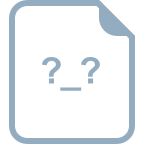
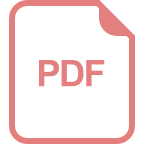
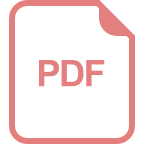















