在uniapp 怎么做js 3d标签云
时间: 2023-11-25 13:08:20 浏览: 102
在uniapp中,你可以使用Three.js来创建3D标签云。以下是大致的步骤:
1. 在uniapp项目中安装Three.js。可以使用npm或下载Three.js的压缩包并将其添加到项目中。
2. 在Vue组件中创建一个canvas元素,并将其作为Three.js渲染器的输出。
3. 在组件的mounted钩子函数中创建Three.js场景并添加相机、灯光和标签云对象。
4. 在组件的updated钩子函数中更新标签云对象的位置和旋转角度。
5. 在组件的beforeDestroy钩子函数中清除Three.js场景和所有对象,以避免内存泄漏。
以下是一个简单的示例代码,演示了如何在uniapp中创建一个3D标签云:
```vue
<template>
<canvas ref="canvas"></canvas>
</template>
<script>
import * as THREE from 'three';
export default {
data() {
return {
tags: [
{ text: 'Hello', weight: 10 },
{ text: 'World', weight: 5 },
{ text: 'Uniapp', weight: 8 },
// ...
],
camera: null,
scene: null,
renderer: null,
cloud: null,
};
},
mounted() {
// 创建Three.js场景和渲染器
this.scene = new THREE.Scene();
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
this.camera.position.z = 500;
this.renderer = new THREE.WebGLRenderer({
canvas: this.$refs.canvas,
alpha: true,
});
// 创建灯光
const light = new THREE.DirectionalLight(0xffffff, 1);
light.position.set(0, 0, 1);
this.scene.add(light);
// 创建标签云对象
const loader = new THREE.FontLoader();
loader.load('/fonts/helvetiker_regular.typeface.json', (font) => {
const geometry = new THREE.BufferGeometry();
const material = new THREE.PointsMaterial({
color: 0xffffff,
size: 20,
});
const positions = [];
const sizes = [];
const texts = [];
for (let i = 0; i < this.tags.length; i++) {
const tag = this.tags[i];
const text = new THREE.TextGeometry(tag.text, {
font,
size: tag.weight * 2,
height: 0,
});
text.computeBoundingBox();
const centerOffset = -0.5 * (text.boundingBox.max.x - text.boundingBox.min.x);
for (let j = 0; j < text.vertices.length; j++) {
positions.push(text.vertices[j].x + centerOffset, text.vertices[j].y, text.vertices[j].z);
sizes.push(tag.weight);
texts.push(tag.text);
}
}
geometry.setAttribute('position', new THREE.Float32BufferAttribute(positions, 3));
geometry.setAttribute('size', new THREE.Float32BufferAttribute(sizes, 1));
geometry.setAttribute('text', new THREE.StringBufferAttribute(texts, 1));
this.cloud = new THREE.Points(geometry, material);
this.scene.add(this.cloud);
});
// 开始渲染循环
this.renderLoop();
},
updated() {
// 更新标签云对象的位置和旋转角度
if (this.cloud) {
const time = Date.now() * 0.001;
for (let i = 0; i < this.tags.length; i++) {
const tag = this.tags[i];
const size = tag.weight * 2;
const index = i * 6;
const x = Math.sin(time + i * 0.5) * 500;
const y = Math.cos(time + i * 0.3) * 300;
const z = Math.sin(time + i * 0.7) * 400;
this.cloud.geometry.attributes.position.setXYZ(
index, x - size, y, z - size);
this.cloud.geometry.attributes.position.setXYZ(
index + 1, x + size, y, z - size);
this.cloud.geometry.attributes.position.setXYZ(
index + 2, x - size, y, z + size);
this.cloud.geometry.attributes.position.setXYZ(
index + 3, x + size, y, z + size);
this.cloud.geometry.attributes.position.setXYZ(
index + 4, x, y - size, z);
this.cloud.geometry.attributes.position.setXYZ(
index + 5, x, y + size, z);
}
this.cloud.geometry.attributes.position.needsUpdate = true;
}
},
beforeDestroy() {
// 清除Three.js场景和所有对象
if (this.scene && this.cloud) {
this.scene.remove(this.cloud);
this.cloud.geometry.dispose();
this.cloud.material.dispose();
this.cloud = null;
this.scene.dispose();
this.scene = null;
}
},
methods: {
renderLoop() {
requestAnimationFrame(this.renderLoop);
this.renderer.render(this.scene, this.camera);
},
},
};
</script>
```
注意,上述代码中使用了一个字体文件(helvetiker_regular.typeface.json),需要将其添加到你的uniapp项目中。你可以从Three.js官方网站上下载该字体文件。
阅读全文
相关推荐
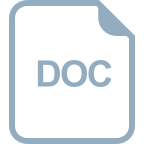
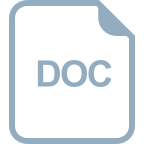
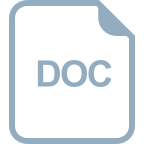
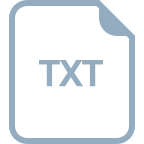
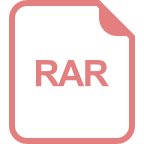
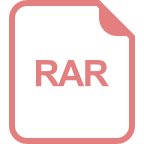
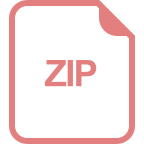
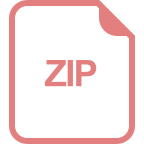
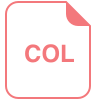







