matlab中三维A*算法
时间: 2023-12-04 09:00:45 浏览: 131
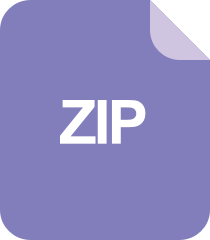
A*算法Matlab实例实现

A*算法是一种在图形搜索和路径规划中广泛应用的启发式搜索算法。它可以用于三维路径规划,但是需要对算法进行适当的修改。
在三维A*算法中,需要将搜索空间从平面扩展到立体空间。这可以通过将每个节点表示为三元组(x,y,z),其中x、y、z分别表示节点在三个轴上的坐标来实现。
在实现A*算法时,需要确定合适的启发式函数来估计从当前节点到目标节点的距离。在三维空间中,可以使用欧几里得距离或曼哈顿距离等距离函数作为启发式函数。
另外,还需要考虑如何表示障碍物。一种常见的方法是将三维空间划分为立方体网格,并将每个网格标记为障碍或非障碍。在搜索过程中,需要避开障碍物。
以下是一个简单的三维A*算法的示例代码:
```
function [path, cost] = astar3d(map, start, goal)
% Three-dimensional A* algorithm
% map: 3D occupancy grid (1: free, 0: occupied)
% start: starting position (3D coordinate)
% goal: goal position (3D coordinate)
% Define heuristic function (Euclidean distance)
heuristic = @(pos) norm(pos - goal);
% Initialize open and closed lists
open = PriorityQueue();
closed = containers.Map();
% Add starting node to open list
g_score = 0;
f_score = g_score + heuristic(start);
open.insert(start, f_score);
% Loop until goal is found or open list is empty
while ~open.isempty()
% Get node with lowest f-score from open list
[current_pos, f_score] = open.pop();
% Check if current node is goal
if isequal(current_pos, goal)
% Reconstruct path and return
path = reconstruct_path(closed, start, goal);
cost = g_score;
return;
end
% Add current node to closed list
closed(num2str(current_pos)) = g_score;
% Expand neighbors
neighbors = get_neighbors(map, current_pos);
for i = 1:size(neighbors, 1)
neighbor_pos = neighbors(i, :);
% Calculate tentative g-score for neighbor
tentative_g_score = g_score + norm(current_pos - neighbor_pos);
% Check if neighbor is already in closed list
if isKey(closed, num2str(neighbor_pos))
% Skip neighbor if it has already been evaluated
continue;
end
% Check if neighbor is in open list
if open.ismember(neighbor_pos)
% Check if tentative g-score is better than previous g-score
if tentative_g_score < closed(num2str(neighbor_pos))
% Update neighbor's g-score and f-score
closed(num2str(neighbor_pos)) = tentative_g_score;
f_score = tentative_g_score + heuristic(neighbor_pos);
open.update(neighbor_pos, f_score);
end
else
% Add neighbor to open list
closed(num2str(neighbor_pos)) = tentative_g_score;
f_score = tentative_g_score + heuristic(neighbor_pos);
open.insert(neighbor_pos, f_score);
end
end
% Update g-score
g_score = closed(num2str(current_pos));
end
% No path found
path = [];
cost = inf;
end
function neighbors = get_neighbors(map, pos)
% Get neighboring nodes that are free and within map bounds
[x, y, z] = ind2sub(size(map), find(map));
neighbors = [x, y, z];
neighbors = neighbors(~ismember(neighbors, pos, 'rows'), :);
distances = pdist2(pos, neighbors);
neighbors(distances > sqrt(3)) = NaN; % limit to 1-neighborhood
neighbors(any(isnan(neighbors), 2), :) = [];
neighbors = neighbors(map(sub2ind(size(map), neighbors(:,1), neighbors(:,2), neighbors(:,3))) == 1, :);
end
function path = reconstruct_path(closed, start, goal)
% Reconstruct path from closed list
path = [goal];
while ~isequal(path(1,:), start)
pos = path(1,:);
for dx = -1:1
for dy = -1:1
for dz = -1:1
neighbor_pos = pos + [dx, dy, dz];
if isKey(closed, num2str(neighbor_pos)) && closed(num2str(neighbor_pos)) < closed(num2str(pos))
pos = neighbor_pos;
end
end
end
end
path = [pos; path];
end
end
classdef PriorityQueue < handle
% Priority queue implemented as binary heap
properties (Access = private)
heap;
count;
end
methods
function obj = PriorityQueue()
obj.heap = {};
obj.count = 0;
end
function insert(obj, item, priority)
% Add item with given priority to queue
obj.count = obj.count + 1;
obj.heap{obj.count} = {item, priority};
obj.sift_up(obj.count);
end
function [item, priority] = pop(obj)
% Remove and return item with lowest priority from queue
item = obj.heap{1}{1};
priority = obj.heap{1}{2};
obj.heap{1} = obj.heap{obj.count};
obj.count = obj.count - 1;
obj.sift_down(1);
end
function update(obj, item, priority)
% Update priority of given item in queue
for i = 1:obj.count
if isequal(obj.heap{i}{1}, item)
obj.heap{i}{2} = priority;
obj.sift_up(i);
break;
end
end
end
function tf = isempty(obj)
% Check if queue is empty
tf = obj.count == 0;
end
function tf = ismember(obj, item)
% Check if item is in queue
tf = false;
for i = 1:obj.count
if isequal(obj.heap{i}{1}, item)
tf = true;
break;
end
end
end
end
methods (Access = private)
function sift_up(obj, index)
% Move item up in heap until it satisfies heap property
while index > 1
parent_index = floor(index / 2);
if obj.heap{index}{2} < obj.heap{parent_index}{2}
temp = obj.heap{index};
obj.heap{index} = obj.heap{parent_index};
obj.heap{parent_index} = temp;
index = parent_index;
else
break;
end
end
end
function sift_down(obj, index)
% Move item down in heap until it satisfies heap property
while index * 2 <= obj.count
child_index = index * 2;
if child_index + 1 <= obj.count && obj.heap{child_index + 1}{2} < obj.heap{child_index}{2}
child_index = child_index + 1;
end
if obj.heap{child_index}{2} < obj.heap{index}{2}
temp = obj.heap{index};
obj.heap{index} = obj.heap{child_index};
obj.heap{child_index} = temp;
index = child_index;
else
break;
end
end
end
end
end
```
该实现使用了一个基于二叉堆的优先队列来管理开放列表,并使用容器映射来管理关闭列表。搜索空间被划分为立方体网格,并使用三元组(x,y,z)表示每个节点的位置。启发式函数使用欧几里得距离,障碍物被标记为0,空闲区域被标记为1。在搜索过程中,只扩展空闲节点,并且避开障碍物。
请注意,该实现并不是最优的实现,因为它没有使用任何优化技巧,如跳跃点或平滑路径。但是,它可以作为三维A*算法的一个简单示例来帮助您开始。
阅读全文
相关推荐
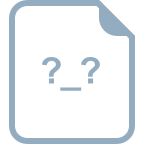
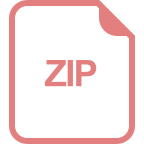
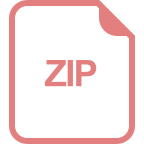
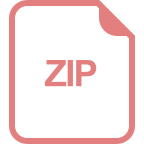
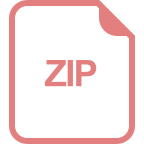
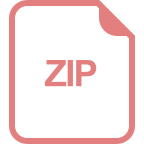
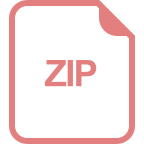
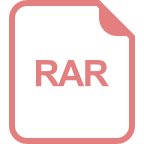
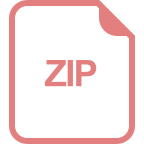
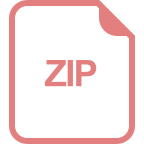
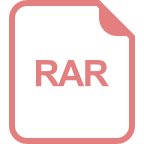
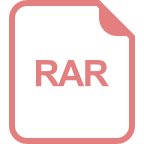