在keil固件库中stm32f103使用矩阵按键控制led亮灭
时间: 2024-04-24 15:27:28 浏览: 9
你可以使用Keil固件库和STM32F103微控制器来实现矩阵按键控制LED的亮灭。以下是一个基本的示例代码:
```c
#include "stm32f10x.h"
#define ROW_NUM 4
#define COL_NUM 4
// 定义矩阵按键的行和列引脚
#define ROW_PORT GPIOA
#define COL_PORT GPIOB
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE); // 使能GPIOC时钟
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13; // PC13
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; // 推挽输出
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; // 最大输出速度50MHz
GPIO_Init(GPIOC, &GPIO_InitStructure); // 初始化GPIOC13
}
void Keypad_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE); // 使能GPIOA和GPIOB时钟
// 配置行引脚为推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置列引脚为上拉输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
uint8_t Keypad_Scan(void)
{
uint8_t row, col;
uint8_t key = 0xFF;
// 遍历每一行
for (row = 0; row < ROW_NUM; row++)
{
// 设置当前行为低电平,其他行为高电平
GPIO_Write(GPIOA, ~(0x01 << row));
// 检测每一列的状态
for (col = 0; col < COL_NUM; col++)
{
if (GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_4 + col) == RESET)
{
// 检测到按键按下
key = (row * COL_NUM) + col;
break;
}
}
if (key != 0xFF)
break;
}
// 恢复所有行引脚为高电平
GPIO_Write(GPIOA, 0xFF);
return key;
}
int main(void)
{
uint8_t key;
LED_Init();
Keypad_Init();
while (1)
{
key = Keypad_Scan();
if (key != 0xFF)
{
// 根据按键值控制LED亮灭
if (key == 0) // 第一行第一列
GPIO_SetBits(GPIOC, GPIO_Pin_13); // 点亮LED
else
GPIO_ResetBits(GPIOC, GPIO_Pin_13); // 熄灭LED
}
}
}
```
这段代码使用了4行4列的矩阵按键,将按键行引脚连接到GPIOA的0至3引脚,将按键列引脚连接到GPIOB的4至7引脚。LED连接到GPIOC的13引脚。在`main`函数中,通过调用`Keypad_Scan`函数来检测按键状态,根据按键值控制LED亮灭。
请注意,以上代码仅供参考,你可能需要根据你的具体硬件连接和需求进行适当的修改。同时,你也需要在Keil中配置正确的编译和调试环境。
相关推荐
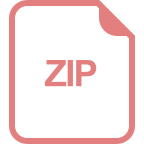
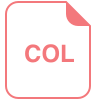
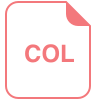
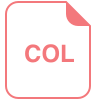
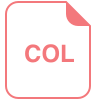
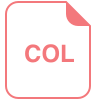









