如何使用sping data neo4j 快速实时插入大量节点数据?
时间: 2024-03-19 08:43:27 浏览: 271
使用Spring Data Neo4j可以通过以下步骤快速实时插入大量节点数据:
1. 配置Neo4j数据库连接:在application.properties文件中添加Neo4j数据库连接信息。
2. 创建节点实体类:使用@NodeEntity注解创建节点实体类,并在类中定义节点属性。
3. 创建节点仓库:使用@NodeRepository注解创建节点仓库,并继承Neo4jRepository接口。
4. 使用Neo4jTemplate插入数据:使用Neo4jTemplate的save方法插入节点数据。可以使用batch方法批量插入数据。
示例代码如下:
```java
// 配置Neo4j数据库连接
@Configuration
@EnableNeo4jRepositories(basePackages = "com.example.repository")
public class Neo4jConfig extends Neo4jConfiguration {
@Bean
public SessionFactory getSessionFactory() {
return new SessionFactory(configuration(), "com.example.entity");
}
@Bean
public org.neo4j.ogm.config.Configuration configuration() {
org.neo4j.ogm.config.Configuration configuration = new org.neo4j.ogm.config.Configuration.Builder()
.uri("bolt://localhost")
.credentials("neo4j", "password")
.build();
return configuration;
}
}
// 创建节点实体类
@NodeEntity
public class Person {
@Id
@GeneratedValue
private Long id;
private String name;
// getters and setters
}
// 创建节点仓库
@Repository
public interface PersonRepository extends Neo4jRepository<Person, Long> {
}
// 插入数据
@Service
public class PersonService {
@Autowired
private Neo4jTemplate neo4jTemplate;
@Autowired
private PersonRepository personRepository;
public void savePerson(Person person) {
// 使用Neo4jTemplate插入数据
neo4jTemplate.save(person);
// 批量插入数据
List<Person> persons = new ArrayList<>();
for (int i = 0; i < 10000; i++) {
Person p = new Person();
p.setName("person" + i);
persons.add(p);
}
neo4jTemplate.batchSave(persons);
}
}
```
以上是一个简单的示例,你可以按照自己的需求进行适当的修改和扩展。
阅读全文
相关推荐
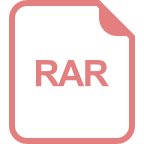
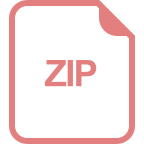
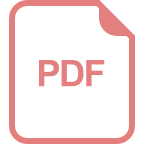















