通过java实现根据封装的知识,编写程序,题目要求: 某汽车销售公司,销售各种小车,有品牌,型号,座位数,颜色,单价,上个月销售量等属性,及计算月销售金额方法。 要求:(1)创建 Car 类封装上述属性与方法。 (2)创建测试类,实例化多部小车,键盘输入数据如图数据,输出相应内容
时间: 2023-05-20 22:07:31 浏览: 45
回答:非常感谢您的问题,以下是针对您提供的题目所编写的Java代码:
```
public class Car {
private String brand;
private String model;
private int seats;
private String color;
private double price;
private int lastMonthSales;
public Car(String brand, String model, int seats, String color, double price, int lastMonthSales) {
this.brand = brand;
this.model = model;
this.seats = seats;
this.color = color;
this.price = price;
this.lastMonthSales = lastMonthSales;
}
public double calculateMonthlySales() {
return price * lastMonthSales;
}
public String toString() {
return "Brand: " + brand + "\nModel: " + model + "\nSeats: " + seats
+ "\nColor: " + color + "\nPrice: " + price + "\nLast month sales: " + lastMonthSales;
}
}
import java.util.Scanner;
public class CarTest {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the number of cars to add: ");
int numCars = scanner.nextInt();
scanner.nextLine();
Car[] cars = new Car[numCars];
for (int i = 0; i < numCars; i++) {
System.out.println("*** Enter car #" + (i + 1) + " details ***");
System.out.print("Brand: ");
String brand = scanner.nextLine();
System.out.print("Model: ");
String model = scanner.nextLine();
System.out.print("Seats: ");
int seats = scanner.nextInt();
scanner.nextLine(); // consume newline left-over
System.out.print("Color: ");
String color = scanner.nextLine();
System.out.print("Price: ");
double price = scanner.nextDouble();
scanner.nextLine(); // consume newline left-over
System.out.print("Last month sales: ");
int lastMonthSales = scanner.nextInt();
scanner.nextLine(); // consume newline left-over
cars[i] = new Car(brand, model, seats, color, price, lastMonthSales);
System.out.println("*** Car added! ***");
}
for (int i = 0; i < numCars; i++) {
System.out.println("\n*** Car #" + (i + 1) + " details ***");
System.out.println(cars[i].toString());
System.out.println("Monthly sales: " + cars[i].calculateMonthlySales());
}
}
}
```
您可以在控制台输入不同的汽车信息,程序会按要求求出每辆汽车的月销售额并输出。请注意代码中的注释以获得更好的理解。
相关推荐
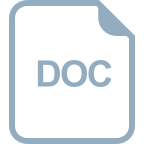
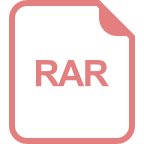
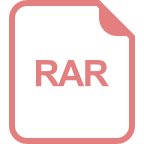















