import com.yy.pojo.Movie; import com.yy.pojo.MovieType; import com.yy.service.Movie.MovieService; import com.yy.service.type.MovieTypeService; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import javax.annotation.Resource; import javax.servlet.http.HttpSession; import java.util.List; @Controller public class MovieController { @Resource private MovieService movieService; @Resource private MovieTypeService movieTypeService; @RequestMapping("/getMovie") public String getMovie(Model model,String name,String type,HttpSession session){ List<MovieType> movieTypeList = movieTypeService.getMovieTypeList(); List<Movie> movieList = movieService.getMovieByNameOrType(name, type); session.setAttribute("movieTypeList",movieTypeList); model.addAttribute("movieList",movieList); return "movie"; } @RequestMapping("/movieList.html") public String getMovieList(Model model, HttpSession session){ List<Movie> movieList = movieService.getMovieList(); List<MovieType> movieTypeList = movieTypeService.getMovieTypeList(); session.setAttribute("movieTypeList",movieTypeList); model.addAttribute("movieList",movieList); return "movie"; } @RequestMapping("/updateMovie") public String updateMovie(Model model, Movie movie){ movieService.updateMovie(movie); Movie movies = movieService.getMovie(movie.getId()); model.addAttribute("movies", movies); return "redirect:/movieList.html"; } @RequestMapping("/toUpdateMoviePage/{movie.id}") public String toUpdate(Model model,@PathVariable("movie.id")Integer id){ model.addAttribute("id",id); return "updateMovie"; } @RequestMapping("/delMovie/{movie.id}") public String deleteMovie(@PathVariable("movie.id")Integer id){ System.out.println(id); movieService.deleteMovieById(id); return "redirect:/movieList.html"; } }解释这段代码,并适当添加注释
时间: 2024-01-30 19:03:41 浏览: 67
这段代码是一个基于 Spring 框架的 Java Web 应用中的控制器类,用于处理与电影相关的请求。具体的功能如下:
1. 导入了一些依赖的类和注解,包括 Movie 和 MovieType 类、MovieService 和 MovieTypeService 接口、Resource、Controller、RequestMapping 等。
2. 使用 @Controller 注解将该类声明为控制器。
3. 使用 @Resource 注解注入了 MovieService 和 MovieTypeService 接口的实现类。
4. 定义了一个处理 "/getMovie" 请求的方法 getMovie,该方法接收名字和类型两个参数,并通过调用 MovieService.getMovieByNameOrType 方法得到对应的电影列表,并将结果存储到 HttpSession 和 Model 中。
5. 定义了一个处理 "/movieList.html" 请求的方法 getMovieList,该方法通过调用 MovieService.getMovieList 方法得到所有电影的列表,并将结果存储到 HttpSession 和 Model 中。
6. 定义了一个处理 "/updateMovie" 请求的方法 updateMovie,该方法接收一个 Movie 对象作为参数,并通过调用 MovieService.updateMovie 方法更新该电影信息,最后将更新后的电影信息存储到 Model 中,并重定向到 "/movieList.html" 页面。
7. 定义了一个处理 "/toUpdateMoviePage/{movie.id}" 请求的方法 toUpdate,该方法接收一个电影 id 作为参数,并将该 id 存储到 Model 中,最后返回 "updateMovie" 页面。
8. 定义了一个处理 "/delMovie/{movie.id}" 请求的方法 deleteMovie,该方法接收一个电影 id 作为参数,并通过调用 MovieService.deleteMovieById 方法删除该电影信息,最后重定向到 "/movieList.html" 页面。
9. 代码中添加了适当的注释,方便理解代码逻辑和功能。
阅读全文
相关推荐
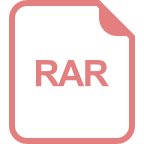
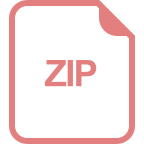
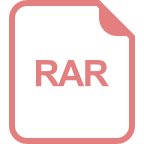








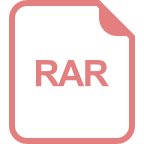
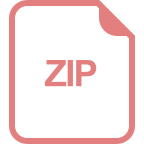
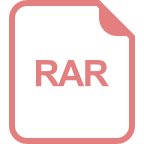
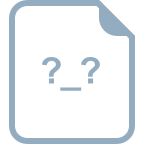
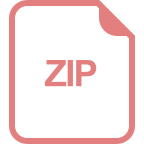
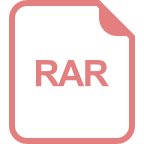
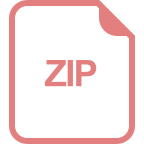