#include <stdio.h> int main() { double a, b; scanf("%lg%lg", &a, &b); printf("%g %g\n", a, b); printf("%g %g\n", a, b); return 0; } void RealSwap(double *x, double *y) { double t = *x; }
时间: 2024-01-13 14:05:47 浏览: 6
您的代码似乎不完整,RealSwap函数只有一句话,没有实现交换x和y的值的操作。如果要实现交换操作,可以在RealSwap函数中添加以下代码:
```
double t = *x;
*x = *y;
*y = t;
```
这样就可以实现交换x和y的值。
相关问题
#include <stdio.h> int main() { double a, b; scanf("%lg%lg", &a, &b); printf("%g %g\n", a, b); printf("%g %g\n", a, b); return 0; }
这段代码的功能是读取两个浮点数,然后输出两遍这两个浮点数。具体来说,程序首先使用 `scanf()` 函数读取两个浮点数,然后使用 `printf()` 函数连续输出两行,每行输出两个浮点数,中间用空格隔开。最后返回 0,表示程序正常结束。
需要注意的是,这里使用 `%lg` 作为 `scanf()` 函数的格式控制字符串,这个格式控制字符串可以读取科学计数法表示的浮点数,例如 `1.23e-4`。而在 `printf()` 函数中,可以使用 `%g` 来输出浮点数,这个格式控制字符串会自动选择最短的表示方法,例如 `0.00123`。
求两点间的距离#include <stdio.h> #include <math.h> typedef struct { double x, y; } POINT; int main() { POINT a, b; double s, p, q; scanf("%lf %lf", &a.x, &a.y); scanf("%lf %lf", &b.x, &b.y); printf("%g\n", s);
你可以使用勾股定理求解两点之间的距离,具体代码如下:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x, y;
} POINT;
int main() {
POINT a, b;
double s, p, q;
scanf("%lf %lf", &a.x, &a.y);
scanf("%lf %lf", &b.x, &b.y);
p = a.x - b.x;
q = a.y - b.y;
s = sqrt(p * p + q * q);
printf("%g\n", s);
return 0;
}
```
其中,p和q分别代表两个点在x轴和y轴上的坐标差值,s为两点之间的距离,使用了数学库中的sqrt函数来求解根号。
阅读全文
相关推荐
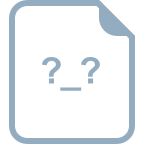












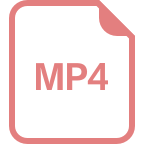
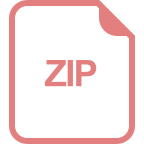
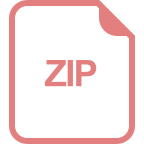