写一套c++ opencv的焊缝提取代码
时间: 2023-03-19 19:24:29 浏览: 336
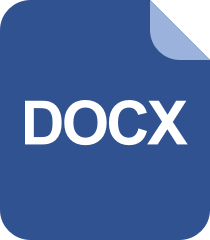
opencv特征提取的代码
以下是一个简单的基于OpenCV的焊缝提取代码示例,其中使用C ++编写。该代码可用于检测由边缘检测器检测到的焊缝边缘。
首先,需要包含OpenCV的头文件,然后加载待处理图像并进行灰度转换和高斯滤波:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main(int argc, char** argv)
{
// Load image
Mat image = imread("welding.jpg");
// Convert to grayscale
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY);
// Apply Gaussian blur
Mat blurred;
GaussianBlur(gray, blurred, Size(3, 3), 0);
```
接下来,使用Canny边缘检测器检测焊缝边缘:
```
// Detect edges using Canny
Mat edges;
Canny(blurred, edges, 50, 150);
```
然后,使用霍夫变换检测直线,这些直线可能是焊缝的边缘:
```
// Detect lines using Hough transform
vector<Vec2f> lines;
HoughLines(edges, lines, 1, CV_PI / 180, 100);
// Draw lines on original image
Mat result = image.clone();
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0], theta = lines[i][1];
Point pt1, pt2;
double a = cos(theta), b = sin(theta);
double x0 = a * rho, y0 = b * rho;
pt1.x = cvRound(x0 + 1000 * (-b));
pt1.y = cvRound(y0 + 1000 * (a));
pt2.x = cvRound(x0 - 1000 * (-b));
pt2.y = cvRound(y0 - 1000 * (a));
line(result, pt1, pt2, Scalar(0, 0, 255), 3, LINE_AA);
}
// Display result
imshow("Welding Seam Detection", result);
waitKey(0);
return 0;
}
```
代码将检测到的线绘制在原始图像上,并在窗口中显示结果。你可以调整Canny边缘检测器的阈值和霍夫变换的参数以获取更好的结果。
阅读全文
相关推荐
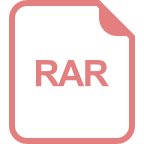
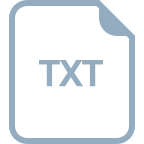
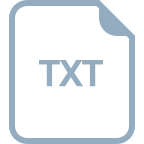
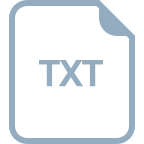
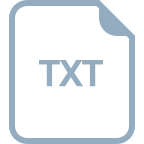
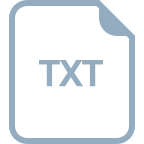
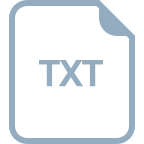
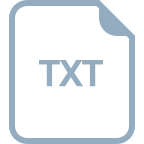
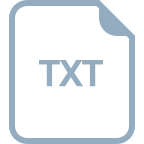
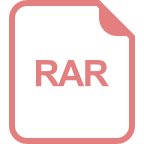
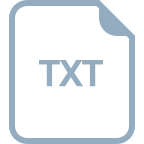
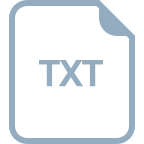
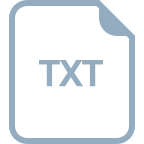