Python中update函数示例
时间: 2023-11-05 21:16:23 浏览: 69
在Python中,update()函数是用来将一个字典类型的数据合并到另一个字典中的函数。举个例子,假设我们有两个字典a和b,我们想将字典b合并到字典a中:
```python
a = {"name": "Tom", "age": 20}
b = {"gender": "male", "height": 175}
a.update(b)
print(a)
```
输出结果为:
```
{'name': 'Tom', 'age': 20, 'gender': 'male', 'height': 175}
```
可以看到,字典b中的键值对被合并到了字典a中。如果字典a中已经存在了某个键,那么它的值会被字典b中对应键的值覆盖。
相关问题
python中update函数示例
在Python中,update()函数是用来将一个字典类型的数据合并到另一个字典中的函数。举个例子,假设我们有两个字典a和b,我们想将字典b合并到字典a中:
```python
a = {"name": "Tom", "age": 20}
b = {"gender": "male", "height": 175}
a.update(b)
print(a)
```
输出结果为:
```
{'name': 'Tom', 'age': 20, 'gender': 'male', 'height': 175}
```
可以看到,字典b中的键值对被合并到了字典a中。如果字典a中已经存在了某个键,那么它的值会被字典b中对应键的值覆盖。
python强化学习代码示例
以下是一个简单的强化学习代码示例,使用Python和Keras实现了一个基本的Q-learning算法:
```python
import random
import numpy as np
from keras.models import Sequential
from keras.layers import Dense
# 定义游戏环境
num_states = 10
num_actions = 4
# 定义Q-learning算法参数
epsilon = 1.0
epsilon_min = 0.01
epsilon_decay = 0.995
gamma = 0.95
learning_rate = 0.001
batch_size = 32
memory = []
# 定义神经网络模型
model = Sequential()
model.add(Dense(24, input_dim=num_states, activation='relu'))
model.add(Dense(24, activation='relu'))
model.add(Dense(num_actions, activation='linear'))
model.compile(loss='mse', optimizer='adam')
# 定义选择动作的函数
def choose_action(state):
if np.random.rand() < epsilon:
return random.randrange(num_actions)
else:
q_values = model.predict(state)
return np.argmax(q_values[0])
# 定义更新模型的函数
def update_model():
if len(memory) < batch_size:
return
batch = random.sample(memory, batch_size)
for state, action, reward, next_state, done in batch:
target = reward
if not done:
target = reward + gamma * np.amax(model.predict(next_state)[0])
target_f = model.predict(state)
target_f[0][action] = target
model.fit(state, target_f, epochs=1, verbose=0)
if epsilon > epsilon_min:
epsilon *= epsilon_decay
# 训练模型
for episode in range(100):
state = np.random.rand(1, num_states)
for step in range(100):
action = choose_action(state)
next_state = np.random.rand(1, num_states)
reward = np.random.rand()
done = False
memory.append((state, action, reward, next_state, done))
state = next_state
update_model()
if done:
break
```
这个示例实现了一个简单的Q-learning算法,使用神经网络模型来估计每个状态-动作对的价值,并使用经验回放来更新模型。在训练过程中,每个episode中的状态是随机生成的,动作是根据当前状态和epsilon-greedy策略选择的,奖励是随机生成的。这个示例只是一个基本的框架,可以根据具体的应用场景进行修改和扩展。
阅读全文
相关推荐
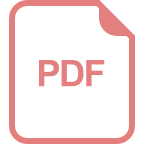
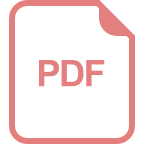
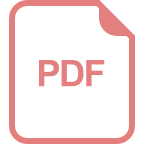
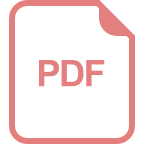
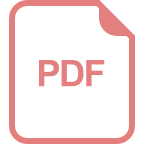
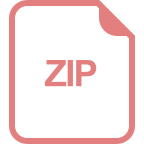
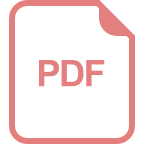
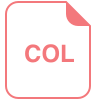
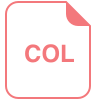






