vue3前端怎么定时发送axios请求
时间: 2023-10-17 09:22:02 浏览: 40
你可以使用JavaScript中的setInterval()函数来定时发送axios请求。setInterval()函数会在指定的时间间隔内重复执行一个指定的函数。
以下是一个示例代码,每隔5秒钟向服务器发送一个axios请求:
```
setInterval(function() {
axios.get('https://example.com/api/data')
.then(function (response) {
// 处理响应数据
console.log(response);
})
.catch(function (error) {
// 处理错误
console.log(error);
});
}, 5000); // 5000毫秒等于5秒钟
```
这个示例使用了axios库来发送请求。你需要先引入axios库并且确保你已经正确配置了axios以与服务器进行通信。
相关问题
vue前端怎么定时发送axios请求
可以使用 JavaScript 中的 `setInterval()` 方法来设置定时器,然后在定时器中发送 Axios 请求。
例如,以下代码每隔 5 秒钟发送一次 Axios 请求:
```javascript
setInterval(() => {
axios.get('/api/data')
.then(response => {
// 处理响应数据
})
.catch(error => {
// 处理错误
});
}, 5000);
```
你可以将上述代码放在 Vue 组件的 `mounted()` 钩子函数中,这样组件加载完成后就会开始定时发送请求。当组件销毁时,应该使用 `clearInterval()` 方法清除定时器,以免内存泄漏。
```javascript
export default {
mounted() {
this.timer = setInterval(() => {
axios.get('/api/data')
.then(response => {
// 处理响应数据
})
.catch(error => {
// 处理错误
});
}, 5000);
},
destroyed() {
clearInterval(this.timer);
}
}
```
只使用vue3前端实现页面定时轮询源码
你可以使用Vue.js提供的定时器方法(setInterval)来实现页面的定时轮询。下面是一个简单的例子:
```html
<template>
<div>
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
data() {
return {
message: ''
}
},
mounted() {
setInterval(() => {
// 这里写你需要轮询的接口地址
axios.get('/api/data')
.then(response => {
this.message = response.data.message
})
.catch(error => {
console.log(error)
})
}, 5000) // 每5秒轮询一次
}
}
</script>
```
在上述代码中,我们通过`setInterval`方法来实现每5秒轮询一次数据。在组件的`mounted`钩子函数中,我们发送一个GET请求到`/api/data`接口地址,然后将返回的数据赋值给组件的`message`属性。这样,在页面中每5秒钟就会自动更新一次数据。
需要注意的是,如果你的接口返回的数据格式不一样,你需要相应地修改代码。此外,在实际项目中,你还需要考虑一些性能和安全性的问题,比如轮询频率、接口地址的安全性等。
相关推荐
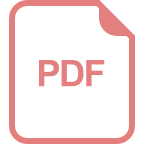
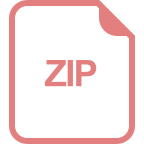
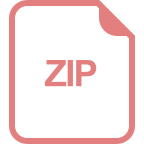












