PCL如何对有序点云中值滤波
时间: 2023-06-20 21:08:18 浏览: 82
在 PCL 中,可以使用 `pcl::MovingLeastSquares` 类来对有序点云进行值滤波。这个类使用最小二乘法拟合一个平滑的曲面,然后将每个点替换为曲面上的对应点。这样可以有效地去除离群点和噪声。
下面是一个简单的示例代码,演示如何使用 `pcl::MovingLeastSquares` 类进行有序点云的值滤波:
```cpp
#include <pcl/point_types.h>
#include <pcl/filters/passthrough.h>
#include <pcl/filters/moving_least_squares.h>
int main(int argc, char** argv)
{
// Load input point cloud
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile("input.pcd", *cloud);
// Filter out points outside a certain range
pcl::PassThrough<pcl::PointXYZ> pass;
pass.setInputCloud(cloud);
pass.setFilterFieldName("z");
pass.setFilterLimits(0.0, 1.0);
pass.filter(*cloud);
// Apply moving least squares filter
pcl::MovingLeastSquares<pcl::PointXYZ, pcl::PointXYZ> mls;
mls.setInputCloud(cloud);
mls.setSearchRadius(0.03);
mls.setPolynomialFit(true);
mls.setPolynomialOrder(2);
mls.setUpsamplingMethod(pcl::MovingLeastSquares<pcl::PointXYZ, pcl::PointXYZ>::SAMPLE_LOCAL_PLANE);
mls.setUpsamplingRadius(0.03);
mls.setUpsamplingStepSize(0.02);
pcl::PointCloud<pcl::PointXYZ>::Ptr filtered_cloud(new pcl::PointCloud<pcl::PointXYZ>);
mls.process(*filtered_cloud);
// Save output point cloud
pcl::io::savePCDFile("output.pcd", *filtered_cloud);
return 0;
}
```
在这个例子中,我们首先使用 `pcl::PassThrough` 类来过滤掉点云中在 z 轴上高度小于 0 或大于 1 的点。然后,我们使用 `pcl::MovingLeastSquares` 类对剩余的点云进行值滤波。我们指定了搜索半径、多项式拟合的阶数和局部平面采样等参数。最后,我们将过滤后的点云保存到文件中。
需要注意的是,`pcl::MovingLeastSquares` 类只适用于有序点云,因为它需要知道每个点的邻域信息。如果你的点云是无序的,可以先使用 `pcl::VoxelGrid` 类将其转换为有序点云。
相关推荐
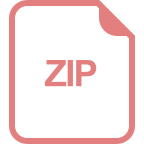
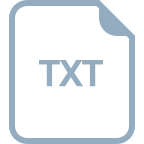














