在C++中使用正则表达式来识别运算符的方法
时间: 2023-06-19 18:05:24 浏览: 64
在C++中,使用正则表达式来识别运算符的方法如下:
1. 引入头文件
```c++
#include <regex>
```
2. 定义正则表达式
```c++
std::regex op_regex("[+\\-*/]");
```
这个正则表达式匹配加、减、乘、除四种运算符。
3. 使用正则表达式进行匹配
```c++
std::string input = "+";
if (std::regex_match(input, op_regex)) {
// input匹配运算符正则表达式
}
```
这里将字符串 `"+”` 与正则表达式进行匹配,如果匹配成功,则进入 if 语句块。
完整代码示例:
```c++
#include <iostream>
#include <regex>
int main() {
std::regex op_regex("[+\\-*/]");
std::string input = "+";
if (std::regex_match(input, op_regex)) {
std::cout << input << " is an operator." << std::endl;
} else {
std::cout << input << " is not an operator." << std::endl;
}
input = "3";
if (std::regex_match(input, op_regex)) {
std::cout << input << " is an operator." << std::endl;
} else {
std::cout << input << " is not an operator." << std::endl;
}
return 0;
}
```
输出结果:
```
+ is an operator.
3 is not an operator.
```
相关推荐
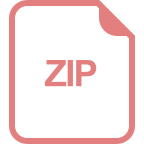














