使用c++编写三角网络五子棋人机对战
时间: 2023-08-17 18:03:59 浏览: 105
三角网络五子棋是一种基于三角形网格的变体五子棋游戏,玩家需要在三角形交汇处落子,而不是在传统的方格交汇处落子。以下是一个基于C++的简单实现:
```c++
#include <iostream>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
const int BOARD_SIZE = 5; // 三角形棋盘大小
const int EMPTY = 0; // 空格子
const int PLAYER_X = 1; // 玩家X方
const int PLAYER_O = 2; // 玩家O方
const int WIN_COUNT = 3; // 获胜所需连续棋子数
// 三角形棋盘类
class TriangularBoard {
public:
TriangularBoard() {
board.resize(BOARD_SIZE);
for (int i = 0; i < BOARD_SIZE; i++) {
board[i].resize(BOARD_SIZE - i);
}
current_player = PLAYER_X;
srand(time(NULL));
}
// 判断当前位置是否可以下子
bool is_valid_move(int row, int col) {
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE - row) {
return false;
}
return board[row][col] == EMPTY;
}
// 下子
void make_move(int row, int col) {
board[row][col] = current_player;
if (current_player == PLAYER_X) {
current_player = PLAYER_O;
} else {
current_player = PLAYER_X;
}
}
// 判断当前状态是否结束
bool is_game_over() {
return get_winner() != EMPTY || get_available_moves().empty();
}
// 获取获胜方
int get_winner() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE - i; j++) {
int winner = get_winner_at_position(i, j);
if (winner != EMPTY) {
return winner;
}
}
}
return EMPTY;
}
// 获取可下子位置列表
vector<pair<int, int>> get_available_moves() {
vector<pair<int, int>> moves;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE - i; j++) {
if (is_valid_move(i, j)) {
moves.push_back(make_pair(i, j));
}
}
}
return moves;
}
// 获取当前玩家
int get_current_player() {
return current_player;
}
// 打印棋盘
void print_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < i; j++) {
cout << " ";
}
for (int j = 0; j < BOARD_SIZE - i; j++) {
if (board[i][j] == PLAYER_X) {
cout << "X ";
} else if (board[i][j] == PLAYER_O) {
cout << "O ";
} else {
cout << ". ";
}
}
cout << endl;
}
}
private:
// 获取指定位置的获胜方
int get_winner_at_position(int row, int col) {
if (board[row][col] == EMPTY) {
return EMPTY;
}
int count1 = 0, count2 = 0, count3 = 0;
int x = row, y = col;
while (x < BOARD_SIZE && y >= 0) {
if (board[x][y] == board[row][col]) {
count1++;
} else {
break;
}
x++;
y--;
}
x = row, y = col;
while (x >= 0 && y < BOARD_SIZE - x) {
if (board[x][y] == board[row][col]) {
count1++;
} else {
break;
}
x--;
y++;
}
x = row, y = col;
while (y < BOARD_SIZE - x) {
if (board[x][y] == board[row][col]) {
count2++;
} else {
break;
}
y++;
}
x = row, y = col;
while (y >= 0) {
if (board[x][y] == board[row][col]) {
count2++;
} else {
break;
}
y--;
}
x = row, y = col;
while (x < BOARD_SIZE) {
if (board[x][y] == board[row][col]) {
count3++;
} else {
break;
}
x++;
}
x = row, y = col;
while (x >= 0) {
if (board[x][y] == board[row][col]) {
count3++;
} else {
break;
}
x--;
}
if (count1 >= WIN_COUNT || count2 >= WIN_COUNT || count3 >= WIN_COUNT) {
return board[row][col];
} else {
return EMPTY;
}
}
vector<vector<int>> board; // 棋盘
int current_player; // 当前玩家
};
// 人机对战类
class HumanVsAI {
public:
HumanVsAI() {
board = TriangularBoard();
srand(time(NULL));
}
// 进行游戏
void play() {
while (!board.is_game_over()) {
board.print_board();
if (board.get_current_player() == PLAYER_X) {
cout << "Your turn (row col): ";
int row, col;
cin >> row >> col;
if (board.is_valid_move(row, col)) {
board.make_move(row, col);
} else {
cout << "Invalid move!" << endl;
}
} else {
cout << "Computer's turn..." << endl;
vector<pair<int, int>> moves = board.get_available_moves();
int index = rand() % moves.size();
board.make_move(moves[index].first, moves[index].second);
}
}
board.print_board();
int winner = board.get_winner();
if (winner == PLAYER_X) {
cout << "You win!" << endl;
} else if (winner == PLAYER_O) {
cout << "Computer wins!" << endl;
} else {
cout << "Tie!" << endl;
}
}
private:
TriangularBoard board; // 三角形棋盘
};
int main() {
HumanVsAI game;
game.play();
return 0;
}
```
该程序实现了一个简单的人机对战,玩家使用行列坐标指定下子位置,计算机随机选择一个可用位置下子。可以根据需要对程序进行扩展,例如增加计算机的智能水平,让它根据当前局面选择最优的下子位置。
阅读全文
相关推荐
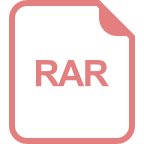
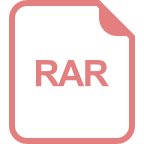
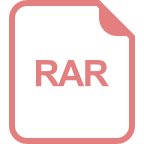
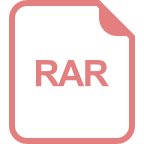
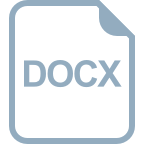
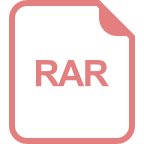
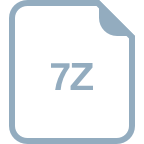
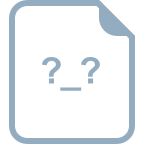
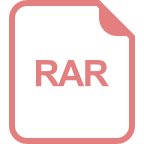
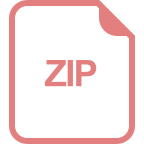
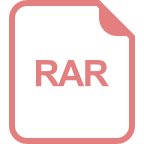