numpy 所有知识点用代码举例出来
时间: 2023-12-03 16:47:46 浏览: 82
好的,以下是一些常见的 NumPy 知识点及其代码示例:
1. 创建数组
```python
import numpy as np
# 创建一维数组
arr1 = np.array([1, 2, 3, 4, 5])
# 创建二维数组
arr2 = np.array([[1, 2, 3], [4, 5, 6]])
# 创建全零数组
zeros = np.zeros((2, 3))
# 创建全一数组
ones = np.ones((2, 3))
# 创建随机数组
random_arr = np.random.rand(2, 3)
```
2. 数组索引和切片
```python
# 一维数组索引
print(arr1[0]) # 1
print(arr1[-1]) # 5
# 二维数组索引
print(arr2[0, 1]) # 2
print(arr2[1, 2]) # 6
# 数组切片
print(arr1[1:4]) # [2, 3, 4]
print(arr2[:, 1:]) # [[2, 3], [5, 6]]
```
3. 数组形状操作
```python
# 获取数组形状
print(arr1.shape) # (5,)
print(arr2.shape) # (2, 3)
# 改变数组形状
arr1_reshape = arr1.reshape((5, 1))
print(arr1_reshape.shape) # (5, 1)
# 数组转置
arr2_T = arr2.T
print(arr2_T.shape) # (3, 2)
```
4. 数组运算
```python
# 数组加法
arr1_plus_one = arr1 + 1
print(arr1_plus_one) # [2, 3, 4, 5, 6]
# 数组乘法
arr2_mul_two = arr2 * 2
print(arr2_mul_two) # [[2, 4, 6], [8, 10, 12]]
# 矩阵乘法
mat1 = np.array([[1, 2], [3, 4]])
mat2 = np.array([[5, 6], [7, 8]])
mat_mul = np.dot(mat1, mat2)
print(mat_mul) # [[19, 22], [43, 50]]
```
以上只是 NumPy 的一些常见知识点,还有很多其他的操作和函数。
阅读全文
相关推荐
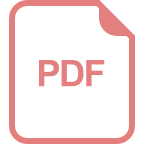
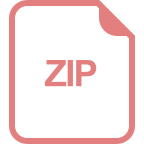
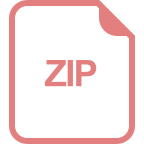
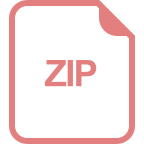
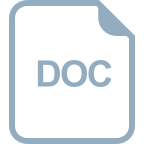
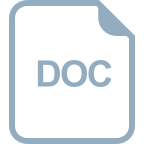
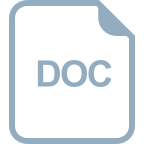
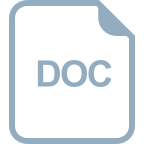
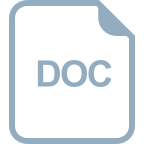
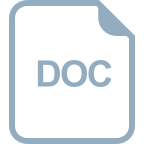
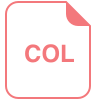
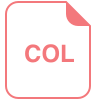
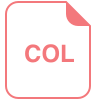
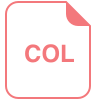
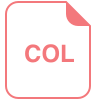
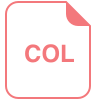
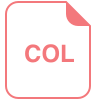
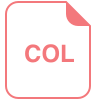