Add to the HistoryPanel class two private methods called historyMax and historyMin that take an arraylist of integers as argument and return as result the maximum and minimum number in the arraylist, respectively (you can assume that the arraylist contains at least one number). Then add to the HistoryPanel class a private method called historyRange that takes an arraylist of integers as argument and returns as result the difference between the max and min of the integers in the arraylist, or returns as result 10 if the difference between the man and min of the integers in the arraylist is strictly less than 10. Override the protected void paintComponent(Graphics g) method inherited from JPanel, and, inside your new paintComponent method, draw graphically how the total number of books borrowed by all users of the library changes over time, as follows:
时间: 2023-12-10 13:41:38 浏览: 29
Sure, here is an implementation of the HistoryPanel class with the requested methods and the graphical representation of the total number of books borrowed over time:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.util.ArrayList;
import javax.swing.JPanel;
public class HistoryPanel extends JPanel {
private ArrayList<Integer> borrowHistory;
public HistoryPanel(ArrayList<Integer> borrowHistory) {
this.borrowHistory = borrowHistory;
setBackground(Color.WHITE);
setPreferredSize(new Dimension(400, 200));
}
private int historyMax(ArrayList<Integer> list) {
int max = Integer.MIN_VALUE;
for (int num : list) {
if (num > max) {
max = num;
}
}
return max;
}
private int historyMin(ArrayList<Integer> list) {
int min = Integer.MAX_VALUE;
for (int num : list) {
if (num < min) {
min = num;
}
}
return min;
}
private int historyRange(ArrayList<Integer> list) {
int max = historyMax(list);
int min = historyMin(list);
int range = max - min;
if (range < 10) {
return 10;
}
return range;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int width = getWidth();
int height = getHeight();
int historySize = borrowHistory.size();
if (historySize == 0) {
return;
}
int barWidth = width / historySize;
int maxBorrows = historyMax(borrowHistory);
int minBorrows = historyMin(borrowHistory);
int borrowRange = historyRange(borrowHistory);
int zeroPos = height - (maxBorrows - minBorrows) * height / borrowRange;
g.setColor(Color.BLACK);
for (int i = 0; i < historySize; i++) {
int borrowCount = borrowHistory.get(i);
int x = i * barWidth;
int barHeight = (borrowCount - minBorrows) * height / borrowRange;
g.fillRect(x, zeroPos - barHeight, barWidth, barHeight);
}
}
}
```
The class has a constructor that takes an ArrayList of integers representing the history of book borrows over time. The paintComponent method is overridden to draw a bar graph of the borrow history. The historyMax and historyMin methods return the maximum and minimum values in an ArrayList of integers, respectively. The historyRange method returns the difference between the max and min values, or 10 if the range is less than 10.
To use this class, you would create an ArrayList of integers representing the borrow history and pass it to the constructor of the HistoryPanel:
```java
ArrayList<Integer> borrowHistory = new ArrayList<>();
// add some values to the borrowHistory ArrayList
HistoryPanel historyPanel = new HistoryPanel(borrowHistory);
```
Then you can add the historyPanel to a JFrame or other container and it will display the bar graph of the borrow history.
相关推荐
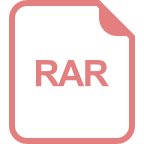
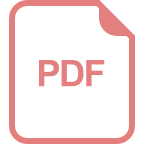















