如何使用Spring Security框架?
时间: 2024-02-22 16:32:22 浏览: 76
Spring Security是一个强大的安全框架,可以在Spring应用程序中提供全面的身份验证和授权机制。下面是使用Spring Security的步骤:
1. 引入Spring Security依赖。可以在Maven或Gradle中添加以下依赖:
```
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.5.0</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.5.0</version>
</dependency>
```
2. 配置Spring Security。创建一个类并添加@Configuration和@EnableWebSecurity注解。在类中重写configure(HttpSecurity http)方法来定义安全规则。
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("ADMIN");
}
}
```
3. 配置用户身份验证。在上面的配置中,我们使用了内存身份验证。但是,在实际应用程序中,我们需要从数据库或LDAP中获取用户信息。可以实现UserDetailsService接口并重写loadUserByUsername方法来提供自定义身份验证。
```
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), getAuthorities(user.getRoles()));
}
private Set<GrantedAuthority> getAuthorities(Set<Role> roles) {
Set<GrantedAuthority> authorities = new HashSet<>();
for (Role role : roles) {
authorities.add(new SimpleGrantedAuthority(role.getName()));
}
return authorities;
}
}
```
4. 集成到应用程序中。可以在Spring应用程序中使用Spring Security来保护不同的URL和资源。可以在Spring MVC控制器中使用@PreAuthorize注解来定义授权规则。
```
@Controller
public class HomeController {
@PreAuthorize("hasRole('ROLE_USER')")
@RequestMapping(value = "/home", method = RequestMethod.GET)
public String home() {
return "home";
}
@PreAuthorize("hasRole('ROLE_ADMIN')")
@RequestMapping(value = "/admin", method = RequestMethod.GET)
public String admin() {
return "admin";
}
}
```
以上就是使用Spring Security框架的基本步骤。当然,Spring Security还有更多高级功能,如OAuth2身份验证等。可以参考官方文档深入学习。
阅读全文
相关推荐
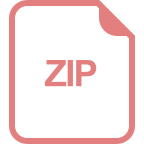
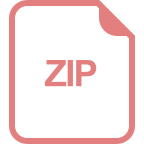
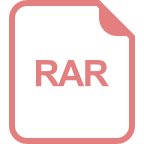
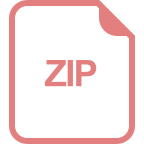
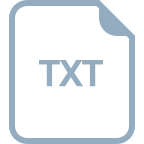
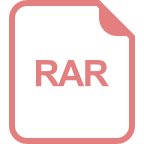
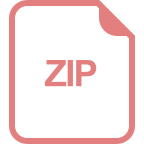
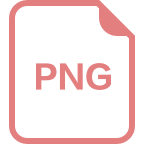
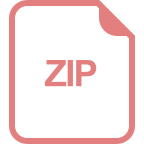
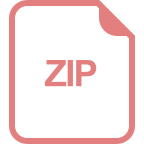
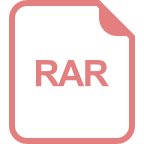
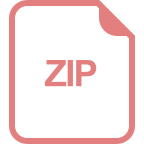
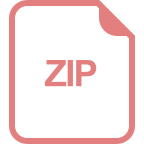
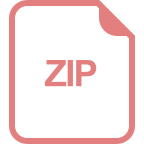
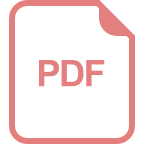
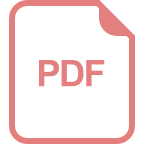
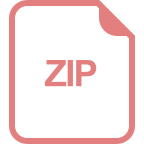