用Pysimplegui写一个车牌识别系统的代码
时间: 2023-12-31 12:04:09 浏览: 26
好的,以下是一个简单的车牌识别系统的Pysimplegui代码示例:
```python
import PySimpleGUI as sg
import cv2
import pytesseract
sg.theme('DarkAmber')
# 定义GUI布局
layout = [[sg.Text('车牌识别系统', font=('Helvetica', 20))],
[sg.Image(filename='', key='-IMAGE-')],
[sg.Button('打开图片'), sg.Button('识别车牌'), sg.Button('退出')]]
window = sg.Window('车牌识别系统', layout)
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == '退出':
break
elif event == '打开图片':
filename = sg.popup_get_file('选择要打开的图片', no_window=True)
if filename:
img = cv2.imread(filename)
window['-IMAGE-'].update(filename=filename)
elif event == '识别车牌':
if 'img' in locals():
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
canny = cv2.Canny(blur, 100, 200)
contours, hierarchy = cv2.findContours(canny, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10]
screenCnt = None
for c in contours:
peri = cv2.arcLength(c, True)
approx = cv2.approxPolyDP(c, 0.018 * peri, True)
if len(approx) == 4:
screenCnt = approx
break
if screenCnt is None:
sg.popup('未能检测到车牌', title='错误')
else:
mask = np.zeros(gray.shape, np.uint8)
new_image = cv2.drawContours(mask, [screenCnt], 0, 255, -1)
new_image = cv2.bitwise_and(img, img, mask=mask)
(x, y) = np.where(mask == 255)
(x1, y1) = (np.min(x), np.min(y))
(x2, y2) = (np.max(x), np.max(y))
cropped_image = gray[x1:x2 + 1, y1:y2 + 1]
text = pytesseract.image_to_string(cropped_image, lang='eng',
config='--psm 11')
sg.popup('识别到的车牌号码为:\n' + text, title='车牌号码')
else:
sg.popup('请先打开一张图片', title='错误')
window.close()
```
此代码中,我们使用了OpenCV库和Pytesseract库进行图像处理和OCR识别,Pysimplegui库用于创建GUI界面。在程序运行时,GUI窗口会显示一个“打开图片”按钮、一个“识别车牌”按钮和一个空白的图像框,用户可以点击“打开图片”按钮来选择要识别的车牌图片,然后点击“识别车牌”按钮进行识别,最终结果会在弹出窗口中显示。
相关推荐
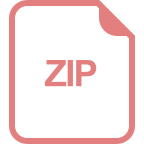
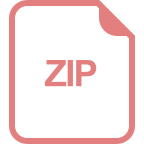
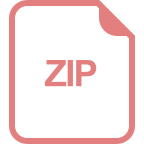














