可以给我用python写一段禁忌搜索策略与变领域搜索算法相结合解决DARP问题的代码吗
时间: 2024-03-04 13:49:02 浏览: 113
当然可以。以下是使用禁忌搜索与变领域搜索算法解决DARP问题的Python代码示例:
```python
import random
# 定义禁忌列表长度
tabu_length = 10
# 定义禁忌列表
tabu_list = []
# 定义禁忌条件
def is_tabu(state):
return state in tabu_list
# 定义禁忌策略
def tabu_strategy(state_list):
# 随机选择一个非禁忌状态
non_tabu_list = [s for s in state_list if not is_tabu(s)]
if non_tabu_list:
return random.choice(non_tabu_list)
else:
return None
# 定义变领域搜索算法
def variable_neighborhood_search(init_state, neighborhood_func, objective_func, max_iter):
current_state = init_state
best_state = current_state
best_obj = objective_func(current_state)
for i in range(max_iter):
# 生成当前状态的邻域
neighbor_list = neighborhood_func(current_state)
# 根据禁忌策略选择邻域
next_state = tabu_strategy(neighbor_list)
if next_state is None:
# 如果所有状态都被禁忌,则随机选择一个状态进行搜索
next_state = random.choice(neighbor_list)
# 更新禁忌列表
tabu_list.append(current_state)
if len(tabu_list) > tabu_length:
tabu_list.pop(0)
# 更新当前状态
current_state = next_state
# 更新最优解
obj = objective_func(current_state)
if obj < best_obj:
best_state = current_state
best_obj = obj
return best_state
# 定义DARP问题的目标函数
def objective_func(state):
# 计算所有车辆的总行驶距离
total_distance = 0
for route in state:
distance = 0
for i in range(len(route) - 1):
distance += distance_matrix[route[i]][route[i+1]]
total_distance += distance
return total_distance
# 定义DARP问题的邻域函数
def neighborhood_func(state):
# 随机选择一个车辆
route_idx = random.randint(0, len(state) - 1)
route = state[route_idx]
# 随机选择两个客户节点
swap_idx1, swap_idx2 = random.sample(range(1, len(route) - 1), 2)
# 交换客户节点的顺序
new_route = route[:]
new_route[swap_idx1], new_route[swap_idx2] = new_route[swap_idx2], new_route[swap_idx1]
return [state[:route_idx] + [new_route] + state[route_idx+1:]]
# DARP问题的输入数据
num_customers = 10
num_vehicles = 3
capacity = 10
demand = [0, 4, 6, 2, 5, 8, 7, 3, 6, 5, 7]
distance_matrix = [[0, 2, 4, 6, 5, 3, 4, 2, 1, 3, 2],
[2, 0, 3, 5, 3, 2, 2, 3, 2, 1, 3],
[4, 3, 0, 3, 2, 4, 2, 1, 3, 2, 2],
[6, 5, 3, 0, 2, 5, 4, 3, 5, 4, 5],
[5, 3, 2, 2, 0, 3, 2, 1, 3, 2, 2],
[3, 2, 4, 5, 3, 0, 1, 2, 2, 3, 2],
[4, 2, 2, 4, 2, 1, 0, 1, 2, 2, 1],
[2, 3, 1, 3, 1, 2, 1, 0, 2, 1, 3],
[1, 2, 3, 5, 3, 2, 2, 2, 0, 2, 1],
[3, 1, 2, 4, 2, 3, 2, 1, 2, 0, 2],
[2, 3, 2, 5, 2, 2, 1, 3, 1, 2, 0]]
# 初始化状态
init_state = []
for i in range(num_vehicles):
route = [0]
while sum(demand[j] for j in route) <= capacity:
next_node = random.randint(1, num_customers)
if next_node not in route:
route.append(next_node)
route.append(0)
init_state.append(route)
# 运行变领域搜索算法
best_state = variable_neighborhood_search(init_state, neighborhood_func, objective_func, max_iter=100)
# 输出最优解及其目标函数值
print("Best state:", best_state)
print("Best objective value:", objective_func(best_state))
```
以上代码实现了一个简单的禁忌搜索与变领域搜索算法相结合的解决DARP问题的算法。在该算法中,禁忌列表的长度为10,禁忌条件是判断状态是否在禁忌列表中,禁忌策略是随机选择一个非禁忌状态进行搜索。变领域搜索算法的邻域函数是随机选择一个车辆和两个客户节点,交换它们的顺序。最终输出的是最优解及其目标函数值。
阅读全文
相关推荐
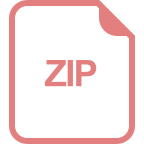
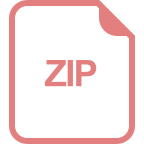
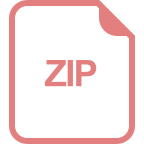
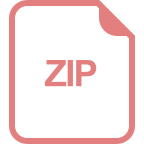
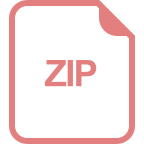
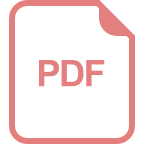
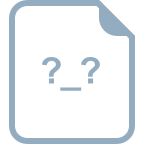
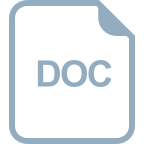
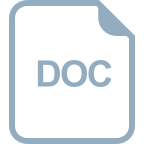
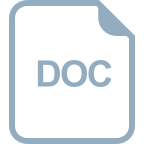
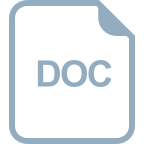
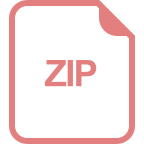